toggleRowSelection vue3
时间: 2023-11-09 07:01:06 浏览: 42
在 Vue 3 中,可以使用 `v-model` 指令和 `isSelected` 属性来实现行的选中和取消选中。具体实现步骤如下:
1. 在表格中的每一行添加一个复选框,并绑定 `v-model` 指令到一个名为 `isSelected` 的 Boolean 类型的变量上。
2. 在表格的 `data` 选项中添加一个名为 `selectedRows` 的数组,用于存储被选中的行的数据。
3. 在复选框的 `change` 事件中,根据 `isSelected` 的值将当前行的数据添加或移除到 `selectedRows` 数组中。
4. 在表格的 `computed` 选项中添加一个名为 `selectedRowKeys` 的计算属性,用于返回被选中行的唯一标识符(例如行的 ID)组成的数组。
5. 在表格的 `props` 选项中添加一个名为 `rowKey` 的属性,用于指定行的唯一标识符字段名。
6. 在表格的 `methods` 选项中添加一个名为 `toggleRowSelection` 的方法,用于切换某一行的选中状态。该方法接受两个参数:行数据和当前行是否被选中。
以下是示例代码:
```html
<template>
<table>
<thead>
<tr>
<th><input type="checkbox" v-model="isAllSelected"></th>
<th>...</th>
</tr>
</thead>
<tbody>
<tr v-for="row in rows" :key="row.id">
<td><input type="checkbox" v-model="row.isSelected"></td>
<td>...</td>
</tr>
</tbody>
</table>
</template>
<script>
export default {
data() {
return {
rows: [
{ id: 1, ... },
{ id: 2, ... },
{ id: 3, ... },
...
],
selectedRows: []
}
},
computed: {
isAllSelected: {
get() {
return this.selectedRows.length === this.rows.length
},
set(value) {
if (value) {
this.selectedRows = this.rows.slice()
} else {
this.selectedRows = []
}
}
},
selectedRowKeys() {
return this.selectedRows.map(row => row[this.rowKey])
}
},
props: {
rowKey: {
type: String,
default: 'id'
}
},
methods: {
toggleRowSelection(row, isSelected) {
if (isSelected) {
this.selectedRows.push(row)
} else {
const index = this.selectedRows.findIndex(selectedRow => selectedRow[this.rowKey] === row[this.rowKey])
if (index !== -1) {
this.selectedRows.splice(index, 1)
}
}
}
}
}
</script>
```
相关推荐
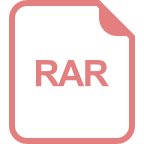














