用python的代码写一个贪吃蛇
时间: 2024-01-06 15:05:08 浏览: 65
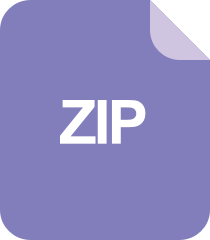
python贪吃蛇完整的源代码资料.zip
好的,以下是一个基于Python的简单贪吃蛇游戏的代码:
```python
import pygame
import random
# 初始化 Pygame
pygame.init()
# 定义颜色
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
# 设置窗口大小
WINDOW_WIDTH = 600
WINDOW_HEIGHT = 400
# 设置游戏窗口
screen = pygame.display.set_mode((WINDOW_WIDTH, WINDOW_HEIGHT))
pygame.display.set_caption("贪吃蛇")
# 设置蛇的初始位置
snake_pos = [100, 50]
snake_body = [[100, 50], [90, 50], [80, 50]]
# 设置食物的初始位置
food_pos = [random.randrange(1, (WINDOW_WIDTH//10)) * 10,
random.randrange(1, (WINDOW_HEIGHT//10)) * 10]
food_spawn = True
# 设置移动方向
direction = "RIGHT"
change_to = direction
# 设置游戏时钟
clock = pygame.time.Clock()
# 设置字体
font_style = pygame.font.SysFont(None, 30)
# 定义游戏结束函数
def game_over():
message = font_style.render("游戏结束!按 Q 退出,按 R 重新开始!", True, RED)
screen.blit(message, [WINDOW_WIDTH/6, WINDOW_HEIGHT/3])
# 游戏循环
while True:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
elif event.type == pygame.KEYDOWN:
if event.key == pygame.K_q:
pygame.quit()
quit()
elif event.key == pygame.K_r:
# 重新开始游戏
snake_pos = [100, 50]
snake_body = [[100, 50], [90, 50], [80, 50]]
food_pos = [random.randrange(1, (WINDOW_WIDTH//10)) * 10,
random.randrange(1, (WINDOW_HEIGHT//10)) * 10]
food_spawn = True
direction = "RIGHT"
change_to = direction
# 处理移动方向
if change_to == "RIGHT" and direction != "LEFT":
direction = "RIGHT"
elif change_to == "LEFT" and direction != "RIGHT":
direction = "LEFT"
elif change_to == "UP" and direction != "DOWN":
direction = "UP"
elif change_to == "DOWN" and direction != "UP":
direction = "DOWN"
# 移动蛇的身体
if direction == "RIGHT":
snake_pos[0] += 10
elif direction == "LEFT":
snake_pos[0] -= 10
elif direction == "UP":
snake_pos[1] -= 10
elif direction == "DOWN":
snake_pos[1] += 10
# 记录蛇的身体
snake_body.insert(0, list(snake_pos))
if snake_pos == food_pos:
food_spawn = False
else:
snake_body.pop()
# 生成新的食物
if not food_spawn:
food_pos = [random.randrange(1, (WINDOW_WIDTH//10)) * 10,
random.randrange(1, (WINDOW_HEIGHT//10)) * 10]
food_spawn = True
# 设置窗口背景颜色
screen.fill(BLACK)
# 绘制蛇和食物
for pos in snake_body:
pygame.draw.rect(screen, GREEN, pygame.Rect(
pos[0], pos[1], 10, 10))
pygame.draw.rect(screen, BLUE, pygame.Rect(
food_pos[0], food_pos[1], 10, 10))
# 检查游戏是否结束
if snake_pos[0] < 0 or snake_pos[0] > WINDOW_WIDTH-10:
game_over()
if snake_pos[1] < 0 or snake_pos[1] > WINDOW_HEIGHT-10:
game_over()
for block in snake_body[1:]:
if snake_pos == block:
game_over()
# 显示分数
score = len(snake_body)-3
score_text = font_style.render("得分: " + str(score), True, WHITE)
screen.blit(score_text, [0, 0])
# 更新屏幕
pygame.display.update()
# 设置帧率
clock.tick(15)
# 获取键盘输入
key = pygame.key.get_pressed()
# 处理键盘输入
if key[pygame.K_RIGHT]:
change_to = "RIGHT"
elif key[pygame.K_LEFT]:
change_to = "LEFT"
elif key[pygame.K_UP]:
change_to = "UP"
elif key[pygame.K_DOWN]:
change_to = "DOWN"
```
运行以上代码,即可开始贪吃蛇游戏。
阅读全文
相关推荐
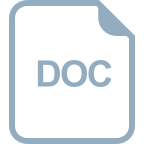
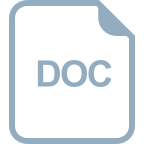












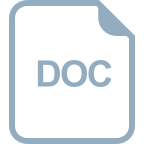
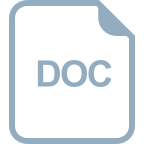
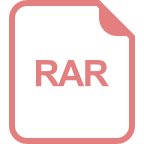