python opencv 模板匹配代码
时间: 2024-12-07 09:12:49 浏览: 22
模板匹配是一种在图像中查找模板图像位置的方法。OpenCV 提供了 `cv2.matchTemplate()` 函数来实现模板匹配。以下是一个简单的示例代码,展示了如何使用 OpenCV 进行模板匹配:
```python
import cv2
import numpy as np
# 读取主图像和模板图像
main_image = cv2.imread('main_image.jpg', cv2.IMREAD_COLOR)
template = cv2.imread('template.jpg', cv2.IMREAD_COLOR)
# 获取模板图像的尺寸
template_height, template_width = template.shape[:2]
# 执行模板匹配
result = cv2.matchTemplate(main_image, template, cv2.TM_CCOEFF_NORMED)
# 设置匹配阈值
threshold = 0.8
loc = np.where(result >= threshold)
# 在匹配位置绘制矩形框
for pt in zip(*loc[::-1]):
cv2.rectangle(main_image, pt, (pt[0] + template_width, pt[1] + template_height), (0, 0, 255), 2)
# 显示结果图像
cv2.imshow('Detected', main_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
### 代码解释
1. **导入库**:
```python
import cv2
import numpy as np
```
导入 OpenCV 和 NumPy 库。
2. **读取图像**:
```python
main_image = cv2.imread('main_image.jpg', cv2.IMREAD_COLOR)
template = cv2.imread('template.jpg', cv2.IMREAD_COLOR)
```
读取主图像和模板图像。
3. **获取模板尺寸**:
```python
template_height, template_width = template.shape[:2]
```
获取模板图像的高度和宽度。
4. **执行模板匹配**:
```python
result = cv2.matchTemplate(main_image, template, cv2.TM_CCOEFF_NORMED)
```
使用 `cv2.matchTemplate()` 函数进行模板匹配。
5. **设置匹配阈值并找到匹配位置**:
```python
threshold = 0.8
loc = np.where(result >= threshold)
```
设置匹配阈值,并找到所有匹配位置。
6. **绘制矩形框**:
```python
for pt in zip(*loc[::-1]):
cv2.rectangle(main_image, pt, (pt[0] + template_width, pt[1] + template_height), (0, 0, 255), 2)
```
在匹配位置绘制红色矩形框。
7. **显示结果图像**:
```python
cv2.imshow('Detected', main_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
显示结果图像,并等待用户按键关闭窗口。
阅读全文
相关推荐
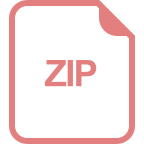
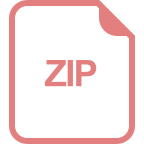
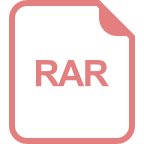















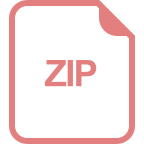