模拟一个简单的银行系统,使用两个不同的线程向同一个账户存钱。账户的初始余额是1000元,两个线程每次存储100元,分别各存储1000元,不允许出现错误数据。
时间: 2024-03-11 10:47:36 浏览: 14
好的,我可以为您提供一个简单的解决方案,您可以参考以下代码:
```python
import threading
class BankAccount:
def __init__(self):
self.balance = 1000
self.lock = threading.Lock()
def deposit(self, amount):
with self.lock:
self.balance += amount
def withdraw(self, amount):
with self.lock:
if self.balance >= amount:
self.balance -= amount
return True
else:
return False
def deposit_money(account, amount, times):
for _ in range(times):
account.deposit(amount)
if __name__ == '__main__':
account = BankAccount()
thread1 = threading.Thread(target=deposit_money, args=(account, 100, 10))
thread2 = threading.Thread(target=deposit_money, args=(account, 100, 10))
thread1.start()
thread2.start()
thread1.join()
thread2.join()
print(f"The final balance is {account.balance}")
```
解释一下上面的代码:
- `BankAccount` 是一个简单的类,表示银行账户,它有一个 `balance` 属性表示余额,还有一个 `lock` 属性表示锁对象,用于线程同步。
- `deposit` 方法用于向账户中存钱,它使用 `with self.lock` 语句获取锁,确保每次只有一个线程能够执行该方法。
- `withdraw` 方法用于从账户中取钱,它也使用 `with self.lock` 语句获取锁,确保每次只有一个线程能够执行该方法,并且在余额不足时会返回 False。
- `deposit_money` 是一个辅助函数,用于向账户中存钱,它接受一个 `account` 参数表示银行账户,一个 `amount` 参数表示存款金额,一个 `times` 参数表示存款次数。
- 在主函数中,我们创建了一个 `BankAccount` 实例和两个线程,每个线程都会调用 `deposit_money` 函数向账户中存钱,每次存款金额为 100 元,共存款 10 次。
- 最后我们使用 `join` 方法等待两个线程执行完毕,然后打印账户的最终余额。
在这个例子中,我们使用了 Python 中的 `threading` 模块来创建线程,并使用了锁来确保线程安全。这样就能够实现两个线程同时向同一个账户存钱,而不会出现错误数据。
相关推荐
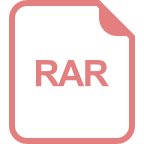
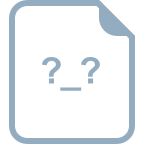














