怎么从tushare提取数据
时间: 2023-08-07 08:08:23 浏览: 40
首先,你需要安装tushare库,可以使用以下命令进行安装:
```
pip install tushare
```
然后,你需要在tushare的官网上注册并获取你的token,用于调用API接口。接下来,你可以使用以下代码从tushare提取数据:
```python
import tushare as ts
# 设置tushare的token
ts.set_token('your_token_here')
# 初始化pro接口
pro = ts.pro_api()
# 获取股票日线数据
df = pro.daily(ts_code='000001.SZ', start_date='20210101', end_date='20210201')
# 打印数据
print(df)
```
上述代码演示了如何获取某只股票在2021年1月1日至2021年2月1日之间的日线数据。你可以根据自己的需求修改代码中的参数。
相关问题
如何通过tushare提取股票的市盈率和净利润同比增长率
您可以使用tushare中的get_profit_data()函数来提取股票的净利润同比增长率,使用get_stock_basics()函数来获取股票的市盈率数据。具体的代码实现如下:
```
import tushare as ts
# 获取股票的市盈率数据
stock_basics = ts.get_stock_basics()
pe_ratio = stock_basics['pe']
# 获取股票的净利润同比增长率数据
profit_data = ts.get_profit_data()
profit_growth_rate = profit_data['net_profit_ratio']
# 打印数据
print("市盈率数据:\n", pe_ratio)
print("净利润同比增长率数据:\n", profit_growth_rate)
```
使用stock_basic从tushare获取股票数据,从中提取股票代码,用此股票代码下载股票交易数据,打印总股票数量,下载花费时间,发送信号股票的下载进度
以下是使用stock_basic从tushare获取股票数据,提取股票代码并下载股票交易数据的Python代码:
```python
import tushare as ts
import time
import threading
# 获取所有股票代码
pro = ts.pro_api()
data = pro.stock_basic(list_status='L', fields='ts_code')
stock_codes = data['ts_code'].tolist()
# 下载股票交易数据
def download_data(stock_code):
print(f'Downloading data for {stock_code}...')
pro = ts.pro_api()
data = pro.daily(ts_code=stock_code)
print(f'Data for {stock_code} downloaded.')
return data
# 打印总股票数量
print(f'Total number of stocks: {len(stock_codes)}')
# 下载股票数据并计时
start_time = time.time()
threads = []
for stock_code in stock_codes:
t = threading.Thread(target=download_data, args=(stock_code,))
threads.append(t)
t.start()
# 发送股票下载进度信号
while True:
completed_threads = sum([1 for t in threads if not t.is_alive()])
print(f'{completed_threads}/{len(threads)} stocks downloaded.')
if completed_threads == len(threads):
break
time.sleep(5)
# 输出花费时间
end_time = time.time()
print(f'Total time taken to download stock data: {end_time - start_time} seconds.')
```
在上述代码中,我们首先使用`stock_basic`函数从tushare获取所有股票代码,然后遍历每个股票代码,使用`pro.daily`函数下载股票交易数据。在下载数据之前,我们使用`threading`模块创建了多个线程同时下载不同股票的数据,以提高下载速度。此外,我们还使用了一个循环来发送信号,以向用户显示股票数据下载的进度。最后,我们输出了总股票数量和下载花费时间。
请注意,由于tushare API的限制,每分钟最多可以下载200个股票的数据。如果您需要下载大量股票的数据,建议将代码分成多个部分,并在多个时间段内运行代码。
相关推荐
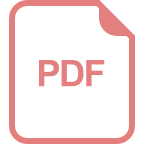











