tinyxml实现xml读取和写入
时间: 2023-09-26 11:07:59 浏览: 221
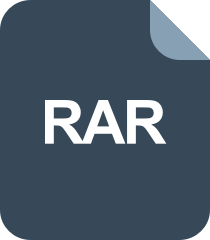
tinyxml读写xml文件

TinyXML是一个轻量级的C++库,用于读取和写入XML文件。以下是一个简单的示例,演示如何使用TinyXML来读取和写入XML文件:
读取XML文件:
```c++
#include "tinyxml.h"
int main()
{
TiXmlDocument doc("example.xml");
bool loadOkay = doc.LoadFile();
if (!loadOkay)
{
printf("Could not load file 'example.xml'. Error='%s'. Exiting.\n", doc.ErrorDesc());
return 1;
}
TiXmlElement* root = doc.FirstChildElement();
if (root == NULL)
{
printf("Failed to load file: No root element.");
doc.Clear();
return 1;
}
TiXmlElement* element = root->FirstChildElement();
while (element != NULL)
{
const char* value = element->Value();
const char* text = element->GetText();
printf("%s: %s\n", value, text);
element = element->NextSiblingElement();
}
doc.Clear();
return 0;
}
```
这个示例假设存在一个名为example.xml的XML文件,并且它有一个根元素和一些子元素。程序读取XML文件并迭代每个子元素,打印其名称和文本内容。
写入XML文件:
```c++
#include "tinyxml.h"
int main()
{
TiXmlDocument doc;
TiXmlDeclaration* decl = new TiXmlDeclaration("1.0", "", "");
doc.LinkEndChild(decl);
TiXmlElement* root = new TiXmlElement("root");
doc.LinkEndChild(root);
TiXmlElement* element = new TiXmlElement("element");
element->LinkEndChild(new TiXmlText("text"));
root->LinkEndChild(element);
doc.SaveFile("example.xml");
return 0;
}
```
这个示例创建了一个新的XML文件,并在其中添加了一个根元素和一个子元素。最后,程序将XML文件保存到名为example.xml的文件中。
阅读全文
相关推荐
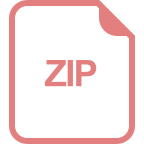
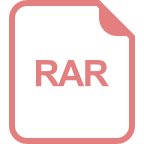
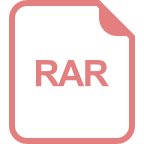
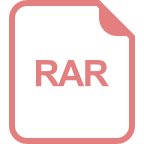
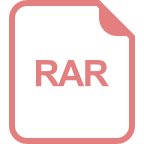
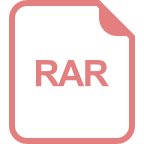
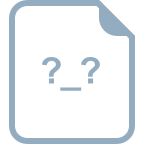
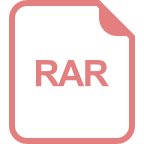
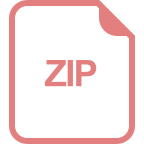
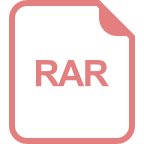
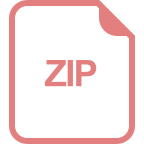
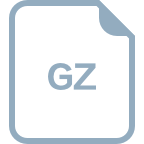
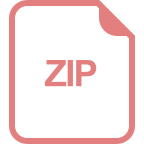
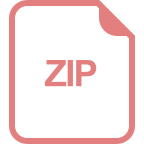
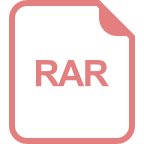
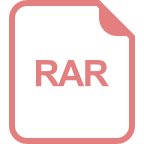