用C++实现一个具有一定功能的计算器,能够进行整数、实数、复数的加、减、乘、除、乘方和求对数等运算。使用时算式采用后缀输入法,每个操作数、操作符之间都以空白符分隔。例如,若要计算3+5则输入3 5 +。乘方运算
时间: 2023-12-28 09:22:33 浏览: 29
可以使用 "^" 符号表示,例如 2 的 3 次方可以表示为 2 3 ^。下面是一个简单的实现示例:
```c++
#include <iostream>
#include <stack>
#include <cmath>
using namespace std;
int main() {
stack<double> numStack;
string input;
while (cin >> input) {
if (input == "+") {
double num2 = numStack.top();
numStack.pop();
double num1 = numStack.top();
numStack.pop();
numStack.push(num1 + num2);
} else if (input == "-") {
double num2 = numStack.top();
numStack.pop();
double num1 = numStack.top();
numStack.pop();
numStack.push(num1 - num2);
} else if (input == "*") {
double num2 = numStack.top();
numStack.pop();
double num1 = numStack.top();
numStack.pop();
numStack.push(num1 * num2);
} else if (input == "/") {
double num2 = numStack.top();
numStack.pop();
double num1 = numStack.top();
numStack.pop();
numStack.push(num1 / num2);
} else if (input == "^") {
double num2 = numStack.top();
numStack.pop();
double num1 = numStack.top();
numStack.pop();
numStack.push(pow(num1, num2));
} else if (input == "log") {
double num = numStack.top();
numStack.pop();
numStack.push(log10(num));
} else if (input == "ln") {
double num = numStack.top();
numStack.pop();
numStack.push(log(num));
} else {
double num = stod(input);
numStack.push(num);
}
}
if (!numStack.empty()) {
cout << numStack.top() << endl;
}
return 0;
}
```
这个实现使用了一个栈来辅助计算,每次读入一个操作数或操作符时,根据其类型进行不同的操作。当最后栈中只剩下一个元素时,即为计算结果。请注意,这个示例中没有处理输入错误的情况,实际应用中需要对输入进行验证和错误处理。
阅读全文
相关推荐





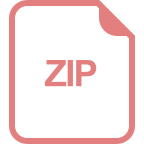
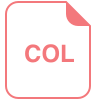
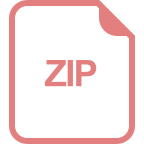
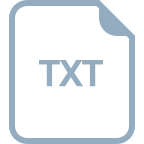
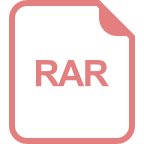
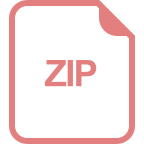