vue 动画 雪花下落 消失 再显示 代码
时间: 2024-05-11 20:15:55 浏览: 109
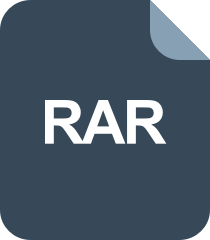
vue实现动画,点击实现隐藏显示
好的,下面是Vue动画实现雪花下落消失再显示的代码:
```
<template>
<div class="snow-container">
<transition-group
:css="false"
name="snow"
mode="out-in"
@before-enter="beforeEnter"
@after-enter="afterEnter"
@before-leave="beforeLeave"
@after-leave="afterLeave"
>
<div
v-for="(snow, index) in snowList"
:key="snow.id"
class="snow"
:style="{ top: snow.y + 'px', left: snow.x + 'px' }"
/>
</transition-group>
</div>
</template>
<script>
const MAX_SNOW_NUM = 20;
const SNOW_SIZE = 10;
export default {
name: 'snow-fall',
data() {
return {
snowList: [] // 雪花列表
};
},
methods: {
// 生成一个随机的雪花实例
createSnow() {
const id = Date.now + Math.round(Math.random() * 100);
const x = Math.round(Math.random() * window.innerWidth);
const y = Math.round(Math.random() * -500);
return { id, x, y };
},
// 添加雪花
addSnow() {
if (this.snowList.length >= MAX_SNOW_NUM) return;
const snow = this.createSnow();
this.snowList.push(snow);
},
// 雪花进入前的动画
beforeEnter(el) {
const delay = Math.round(Math.random() * 2000);
el.style.transitionDelay = delay + 'ms';
el.style.opacity = 0;
},
// 雪花进入后的动画
afterEnter(el) {
el.style.transitionDelay = 0;
const snow = this.snowList.find(s => s.id === el.key);
snow.y = window.innerHeight;
this.$forceUpdate(); // 强制更新Dom
},
// 雪花离开前的动画
beforeLeave(el) {
el.style.opacity = 0;
},
// 雪花离开后的动画
afterLeave(el) {
const snowIndex = this.snowList.findIndex(s => s.id === el.key);
this.snowList.splice(snowIndex, 1);
this.addSnow(); // 添加新的雪花
}
},
mounted() {
for (let i = 0; i < MAX_SNOW_NUM; i++) {
this.addSnow();
}
setInterval(() => {
this.snowList.length < MAX_SNOW_NUM && this.addSnow();
}, 1000);
}
}
</script>
<style scoped>
.snow-container {
position: relative;
width: 100%;
height: 100%;
overflow: hidden;
}
.snow {
position: absolute;
width: ${SNOW_SIZE}px;
height: ${SNOW_SIZE}px;
background-color: #fff;
border-radius: 50%;
opacity: 1;
filter: blur(1px);
pointer-events: none;
z-index: 2;
transition: all 1s ease-in-out;
}
.snow-enter-active,
.snow-leave-active {
opacity: 1;
}
.snow-enter-to,
.snow-leave-from {
opacity: 0;
}
</style>
```
这段代码使用了Vue的动画类库,通过`transition-group`来实现雪花的下落、消失、再显示。具体实现细节可以参考代码注释。
阅读全文
相关推荐
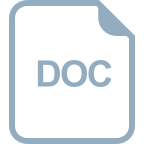
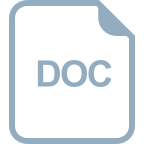
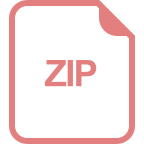
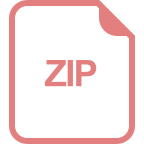
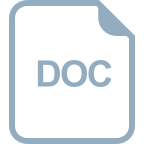
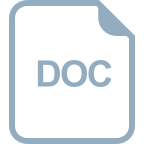



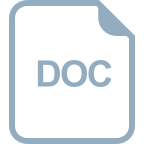
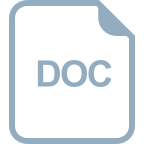