用qt编写代码:恐龙小游戏
时间: 2023-07-27 10:15:40 浏览: 140
以下是一个简单的恐龙小游戏的Qt代码示例,您可以根据需要进行修改和扩展。
```cpp
#include <QApplication>
#include <QGraphicsScene>
#include <QGraphicsView>
#include <QTimer>
#include <QKeyEvent>
#include <QLabel>
#include <QFont>
class Dinosaur : public QObject, public QGraphicsRectItem
{
Q_OBJECT
public:
Dinosaur()
{
setRect(0, 0, 30, 30);
}
void keyPressEvent(QKeyEvent *event)
{
if (event->key() == Qt::Key_Space)
{
jump();
}
}
public slots:
void move()
{
setPos(x() + 10, y());
}
private:
void jump()
{
if (onGround)
{
onGround = false;
QTimer *timer = new QTimer(this);
connect(timer, SIGNAL(timeout()), this, SLOT(fall()));
timer->start(25);
}
}
void fall()
{
setPos(x(), y() + 10);
if (y() >= groundY)
{
setPos(x(), groundY);
onGround = true;
QObject::sender()->deleteLater();
}
}
bool onGround = true;
int groundY = 250;
};
class Obstacle : public QObject, public QGraphicsRectItem
{
Q_OBJECT
public:
Obstacle()
{
setRect(0, 0, 20, 20);
setPos(400, 250);
}
public slots:
void move()
{
setPos(x() - 10, y());
if (x() < -20)
{
QObject::sender()->deleteLater();
}
}
};
class Game : public QGraphicsView
{
public:
Game(QWidget *parent = 0)
: QGraphicsView(parent)
{
scene = new QGraphicsScene(this);
setScene(scene);
setFixedSize(800, 600);
setHorizontalScrollBarPolicy(Qt::ScrollBarAlwaysOff);
setVerticalScrollBarPolicy(Qt::ScrollBarAlwaysOff);
}
void start()
{
scene->setBackgroundBrush(Qt::white);
dinosaur = new Dinosaur();
dinosaur->setPos(50, 250);
scene->addItem(dinosaur);
QTimer *dinoTimer = new QTimer(this);
connect(dinoTimer, SIGNAL(timeout()), dinosaur, SLOT(move()));
dinoTimer->start(50);
QTimer *obstacleTimer = new QTimer(this);
connect(obstacleTimer, SIGNAL(timeout()), this, SLOT(spawnObstacle()));
obstacleTimer->start(2000);
scoreLabel = new QLabel("Score: 0", this);
scoreLabel->setFont(QFont("Arial", 20));
scoreLabel->setGeometry(10, 10, 200, 50);
score = 0;
}
public slots:
void spawnObstacle()
{
Obstacle *obstacle = new Obstacle();
scene->addItem(obstacle);
QTimer *timer = new QTimer(this);
connect(timer, SIGNAL(timeout()), obstacle, SLOT(move()));
timer->start(50);
connect(timer, SIGNAL(timeout()), this, SLOT(updateScore()));
}
void updateScore()
{
score++;
scoreLabel->setText("Score: " + QString::number(score));
}
private:
QGraphicsScene *scene;
Dinosaur *dinosaur;
QLabel *scoreLabel;
int score;
};
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
Game game;
game.show();
game.start();
return a.exec();
}
#include "main.moc"
```
在该示例中,我们创建了一个名为Game的类,它继承自QGraphicsView,它将用于显示游戏场景。在start()函数中,我们为游戏场景添加了一个白色背景、恐龙、定时器和障碍物。恐龙可以通过按下空格键来跳跃,定时器会定期移动恐龙和障碍物,障碍物超出屏幕后会被删除,玩家每成功避开一个障碍物,得分就会增加,分数会显示在屏幕上。
请注意,这只是一个简单的示例,您可以根据需要进行修改和扩展,例如添加更多的障碍物、动画、声音和游戏结束的逻辑。
阅读全文
相关推荐

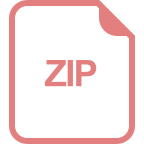
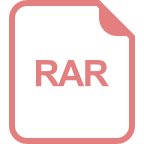
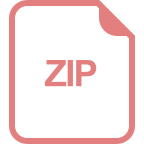
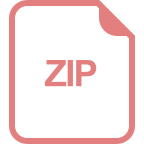
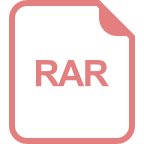
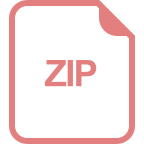
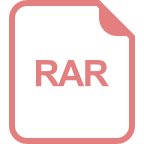
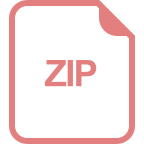
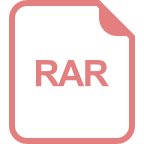
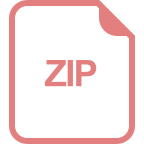
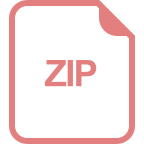
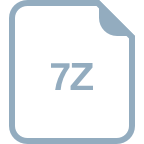