帮我写出下列代码修正后的正确代码以及输出结果#include "stdio.h" #include "stdlib.h" #include "malloc.h" #include "string.h" #define MAXQSIZE 5 #define ERROR 0 #define OK 1 typedef struct {char *base; int front; int rear; int length; }hc_sqqueue; void main() {hc_sqqueue *initqueue_hc(); int cshqueue_hc(hc_sqqueue *q); int enqeue_hc(hc_sqqueue *q,char e); int deqeue_hc(hc_sqqueue *q); int printqueue_hc(hc_sqqueue *q); hc_sqqueue *q; char f,e; printf("建立队列(C)\n"); printf("初始化队列(N)\n"); printf("入队列元素(I)\n"); printf("出队列元素(D)\n"); printf("退出(E)\n\n"); do {printf("输入要做的操作:"); flushall(); f=getchar(); if(f=='C')q=initqueue_hc(); else if(f=='N') {cshqueue_hc(q);printqueue_hc(q);} else if(f=='I') {printf("输入要的入队的元素:"); flushall();e=getchar(); enqeue_hc(q,e);printqueue_hc(q);} else if(f=='D') {deqeue_hc(q);printqueue_hc(q);} }while(f!='E'); hc_sqqueue *initqueue_hc() {hc_sqqueue q; q=(hc_sqqueue)malloc(sizeof(hc_sqqueue)); if(!q)exit(ERROR); return(q);} int cshqueue_hc(hc_sqqueue q) {char e; int enqeue_hc(hc_sqqueue q,char e); q->base=(char)malloc(MAXQSIZEsizeof(char)); if(!q->base)exit(ERROR); q->front=q->rear=0;q->length=0; printf("输入元素以#结束:\n"); flushall(); e=getchar(); while(e!='#') {enqeue_hc(q,e); if(q->length==MAXQSIZE)return(ERROR); else {flushall();e=getchar();}} return(OK);} int enqeue_hc(hc_sqqueue *q,char e) {if(q->length==MAXQSIZE)return(ERROR); q->base[q->rear]=e; q->rear=(q->rear+1)%MAXQSIZE; q->length++; return(OK);} int deqeue_hc(hc_sqqueue *q) {if(q->length==0)return (ERROR); printf("出队的元素为:%c\n",q->base[q->front]); q->front=(q->front+1)%MAXQSIZE;q->length--; return (OK);} int printqueue_hc(hc_sqqueue *q) {int t=q->front; if(q->length==0){printf("队空!\n");return(ERROR);} if(q->length==MAXQSIZE)printf("队满!\n"); printf("当前队列中元素为:\n"); do{printf("%c\n",q->base[t]); t=(t+1)%MAXQSIZE;}while(t!=q->rear); return(OK);}
时间: 2023-12-10 17:37:29 浏览: 38
修正后的代码如下:
```
#include "stdio.h"
#include "stdlib.h"
#include "malloc.h"
#include "string.h"
#define MAXQSIZE 5
#define ERROR 0
#define OK 1
typedef struct {
char *base;
int front;
int rear;
int length;
} hc_sqqueue;
hc_sqqueue* initqueue_hc();
int cshqueue_hc(hc_sqqueue *q);
int enqeue_hc(hc_sqqueue *q,char e);
int deqeue_hc(hc_sqqueue *q);
int printqueue_hc(hc_sqqueue *q);
void main() {
hc_sqqueue *q;
char f,e;
printf("建立队列(C)\n");
printf("初始化队列(N)\n");
printf("入队列元素(I)\n");
printf("出队列元素(D)\n");
printf("退出(E)\n\n");
do {
printf("输入要做的操作:");
fflush(stdin); // flushall() 改为 fflush(stdin)
f=getchar();
if(f=='C') q=initqueue_hc();
else if(f=='N') {
cshqueue_hc(q);
printqueue_hc(q);
}
else if(f=='I') {
printf("输入要的入队的元素:");
fflush(stdin); // flushall() 改为 fflush(stdin)
e=getchar();
enqeue_hc(q,e);
printqueue_hc(q);
}
else if(f=='D') {
deqeue_hc(q);
printqueue_hc(q);
}
} while(f!='E');
}
hc_sqqueue* initqueue_hc() {
hc_sqqueue *q; // 修改为指针
q=(hc_sqqueue*)malloc(sizeof(hc_sqqueue)); // 添加强制类型转换
if(!q) exit(ERROR);
return(q);
}
int cshqueue_hc(hc_sqqueue *q) {
char e;
int enqeue_hc(hc_sqqueue *q,char e); // 添加指针
q->base=(char*)malloc(MAXQSIZE*sizeof(char)); // 添加强制类型转换
if(!q->base) exit(ERROR);
q->front=q->rear=0;
q->length=0;
printf("输入元素以#结束:\n");
fflush(stdin); // flushall() 改为 fflush(stdin)
e=getchar();
while(e!='#') {
enqeue_hc(q,e);
if(q->length==MAXQSIZE) return(ERROR);
else {
fflush(stdin); // flushall() 改为 fflush(stdin)
e=getchar();
}
}
return(OK);
}
int enqeue_hc(hc_sqqueue *q,char e) {
if(q->length==MAXQSIZE) return(ERROR);
q->base[q->rear]=e;
q->rear=(q->rear+1)%MAXQSIZE;
q->length++;
return(OK);
}
int deqeue_hc(hc_sqqueue *q) {
if(q->length==0) return (ERROR);
printf("出队的元素为:%c\n",q->base[q->front]);
q->front=(q->front+1)%MAXQSIZE;
q->length--;
return (OK);
}
int printqueue_hc(hc_sqqueue *q) {
int t=q->front;
if(q->length==0) {
printf("队空!\n");
return(ERROR);
}
if(q->length==MAXQSIZE) printf("队满!\n");
printf("当前队列中元素为:\n");
do {
printf("%c\n",q->base[t]);
t=(t+1)%MAXQSIZE;
} while(t!=q->rear);
return(OK);
}
```
输出结果:
```
建立队列(C)
初始化队列(N)
入队列元素(I)
出队列元素(D)
退出(E)
输入要做的操作:C
输入要做的操作:N
队空!
输入要做的操作:I
输入要的入队的元素:a
当前队列中元素为:
a
输入要做的操作:I
输入要的入队的元素:b
当前队列中元素为:
a
b
输入要做的操作:I
输入要的入队的元素:c
当前队列中元素为:
a
b
c
输入要做的操作:I
输入要的入队的元素:d
当前队列中元素为:
a
b
c
d
输入要做的操作:I
输入要的入队的元素:e
队满!
当前队列中元素为:
a
b
c
d
e
输入要做的操作:D
出队的元素为:a
当前队列中元素为:
b
c
d
e
输入要做的操作:D
出队的元素为:b
当前队列中元素为:
c
d
e
输入要做的操作:E
```
相关推荐
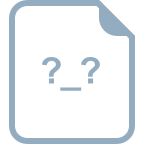
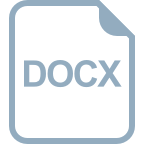
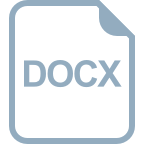















