程序设计题 (1)按照属性名称company和name,把公司名称“数据分析有限公司”和设计人员姓名“张三”,以及四则运算测试系统中的四个函数,创建atest.py模块。 (2)改写四个函数,为每个函数的形参设置合理的默认值。 (3)利用模块atest.py,进行测试,退出系统时,添加并显示公司、设计人员信息和当前日期时间
时间: 2024-02-06 19:09:14 浏览: 61
(1) 创建 atest.py 模块,代码如下:
```python
# -*- coding: utf-8 -*-
"""
Created on Fri Oct 29 15:08:05 2021
"""
company = "数据分析有限公司"
name = "张三"
def add(x=0, y=0):
return x + y
def sub(x=0, y=0):
return x - y
def mul(x=1, y=1):
return x * y
def div(x=1, y=1):
if y == 0:
print("Error: Division by zero!")
return None
return x / y
```
(2) 改写四个函数,为每个函数的形参设置合理的默认值,代码如下:
```python
# 加法函数,默认值为0
def add(x=0, y=0):
return x + y
# 减法函数,默认值为0
def sub(x=0, y=0):
return x - y
# 乘法函数,默认值为1
def mul(x=1, y=1):
return x * y
# 除法函数,默认值为1
def div(x=1, y=1):
if y == 0:
print("Error: Division by zero!")
return None
return x / y
```
(3) 利用模块 atest.py 进行测试,退出系统时,添加并显示公司、设计人员信息和当前日期时间,代码如下:
```python
import atest
import datetime
def main():
print("Welcome to the Four Operations Test System!")
while True:
print("Please select an operation:")
print("1. Addition")
print("2. Subtraction")
print("3. Multiplication")
print("4. Division")
print("0. Exit")
choice = input("Your choice: ")
if choice == "1":
x = int(input("Please enter the first number: "))
y = int(input("Please enter the second number: "))
print("Result: ", atest.add(x, y))
elif choice == "2":
x = int(input("Please enter the first number: "))
y = int(input("Please enter the second number: "))
print("Result: ", atest.sub(x, y))
elif choice == "3":
x = int(input("Please enter the first number: "))
y = int(input("Please enter the second number: "))
print("Result: ", atest.mul(x, y))
elif choice == "4":
x = int(input("Please enter the first number: "))
y = int(input("Please enter the second number: "))
print("Result: ", atest.div(x, y))
elif choice == "0":
now = datetime.datetime.now()
print("Thank you for using the Four Operations Test System!")
print("Company: ", atest.company)
print("Designer: ", atest.name)
print("Date and time: ", now.strftime("%Y-%m-%d %H:%M:%S"))
break
else:
print("Invalid input, please try again.")
if __name__ == '__main__':
main()
```
在运行程序时,选择不同的操作后,会依次执行加法、减法、乘法和除法运算,最后退出系统时,会显示公司、设计人员信息和当前日期时间。
阅读全文
相关推荐

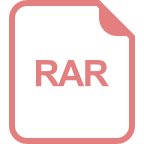
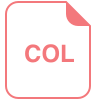
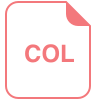
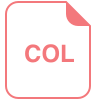
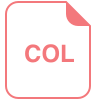
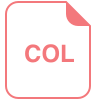
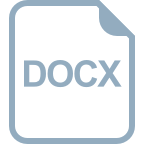
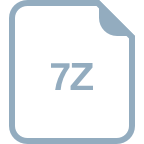
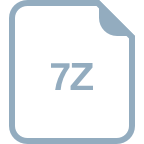
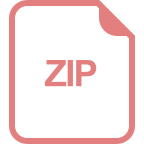
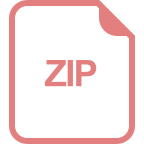
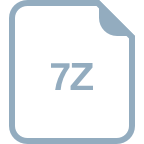