用JAVA语言写出1.生成一个简单文本编辑器,将生成的文本存入文件,如果有打开、存盘、关闭等菜单更佳。GUI界面可以自己灵活设计,同时实现窗体关闭退出功能。 2.编程实现一个加减法计算器。可以使用鼠标来完成加减法
时间: 2024-02-01 21:16:14 浏览: 65
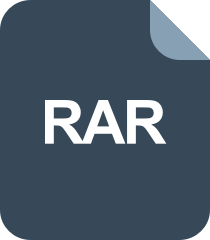
使用java实现复数的加减乘除,并将它们存储在集合中,实现复数的增删改查等,也能按照复数的数学表示格式将集合中所有复数打印输出。
1. 简单文本编辑器
以下是一个基于Java Swing库的简单文本编辑器示例代码:
```
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
import java.io.*;
public class SimpleTextEditor extends JFrame {
private JTextArea textArea;
private JFileChooser fileChooser;
public SimpleTextEditor() {
// 设置窗口标题
super("简单文本编辑器");
// 创建菜单栏
JMenuBar menuBar = new JMenuBar();
// 创建文件菜单
JMenu fileMenu = new JMenu("文件");
JMenuItem openMenuItem = new JMenuItem("打开");
JMenuItem saveMenuItem = new JMenuItem("保存");
JMenuItem exitMenuItem = new JMenuItem("退出");
// 打开文件
openMenuItem.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
int returnVal = fileChooser.showOpenDialog(SimpleTextEditor.this);
if (returnVal == JFileChooser.APPROVE_OPTION) {
File file = fileChooser.getSelectedFile();
try {
FileReader reader = new FileReader(file);
textArea.read(reader, null);
reader.close();
} catch (IOException ex) {
JOptionPane.showMessageDialog(SimpleTextEditor.this, "无法打开文件: " + file.getName(), "错误", JOptionPane.ERROR_MESSAGE);
}
}
}
});
// 保存文件
saveMenuItem.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
int returnVal = fileChooser.showSaveDialog(SimpleTextEditor.this);
if (returnVal == JFileChooser.APPROVE_OPTION) {
File file = fileChooser.getSelectedFile();
try {
FileWriter writer = new FileWriter(file);
textArea.write(writer);
writer.close();
} catch (IOException ex) {
JOptionPane.showMessageDialog(SimpleTextEditor.this, "无法保存文件: " + file.getName(), "错误", JOptionPane.ERROR_MESSAGE);
}
}
}
});
// 退出程序
exitMenuItem.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
System.exit(0);
}
});
// 添加菜单项到菜单
fileMenu.add(openMenuItem);
fileMenu.add(saveMenuItem);
fileMenu.addSeparator();
fileMenu.add(exitMenuItem);
// 添加菜单到菜单栏
menuBar.add(fileMenu);
// 创建文本区域
textArea = new JTextArea();
// 创建文件选择器
fileChooser = new JFileChooser();
fileChooser.setFileFilter(new FileFilter() {
public boolean accept(File file) {
return file.isDirectory() || file.getName().toLowerCase().endsWith(".txt");
}
public String getDescription() {
return "文本文件 (*.txt)";
}
});
// 添加组件到窗口
setJMenuBar(menuBar);
add(new JScrollPane(textArea), BorderLayout.CENTER);
// 设置窗口属性
setSize(600, 400);
setLocationRelativeTo(null);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
public static void main(String[] args) {
SimpleTextEditor editor = new SimpleTextEditor();
editor.setVisible(true);
}
}
```
2. 加减法计算器
以下是一个基于Java Swing库的加减法计算器示例代码:
```
import javax.swing.*;
import java.awt.*;
import java.awt.event.*;
public class Calculator extends JFrame {
private JTextField inputField;
private JButton addButton;
private JButton subtractButton;
private JButton clearButton;
private int result = 0;
public Calculator() {
// 设置窗口标题
super("加减法计算器");
// 创建组件
inputField = new JTextField(10);
addButton = new JButton("+");
subtractButton = new JButton("-");
clearButton = new JButton("清空");
// 添加事件监听器
addButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
try {
int value = Integer.parseInt(inputField.getText());
result += value;
inputField.setText(Integer.toString(result));
} catch (NumberFormatException ex) {
JOptionPane.showMessageDialog(Calculator.this, "请输入一个整数", "错误", JOptionPane.ERROR_MESSAGE);
}
}
});
subtractButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
try {
int value = Integer.parseInt(inputField.getText());
result -= value;
inputField.setText(Integer.toString(result));
} catch (NumberFormatException ex) {
JOptionPane.showMessageDialog(Calculator.this, "请输入一个整数", "错误", JOptionPane.ERROR_MESSAGE);
}
}
});
clearButton.addActionListener(new ActionListener() {
public void actionPerformed(ActionEvent e) {
result = 0;
inputField.setText("");
}
});
// 创建面板并添加组件
JPanel panel = new JPanel();
panel.add(inputField);
panel.add(addButton);
panel.add(subtractButton);
panel.add(clearButton);
// 添加面板到窗口
add(panel);
// 设置窗口属性
pack();
setLocationRelativeTo(null);
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
}
public static void main(String[] args) {
Calculator calculator = new Calculator();
calculator.setVisible(true);
}
}
```
阅读全文
相关推荐
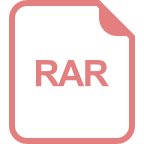













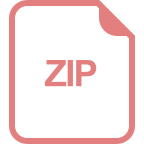