用 C++实现使用 vector 求指定字符串
时间: 2024-04-29 15:26:07 浏览: 17
的出现次数该怎么做?
这个问题涉及到字符串查找和计数,可以使用C语言的标准库函数strstr和strchr来查找子串,再使用一个计数器变量记录出现次数即可。具体实现方法如下:
1. 定义一个计数器变量count,初始值为0。
2. 使用strchr函数查找指定字符串中第一个出现的字符,找到后用strstr函数判断是否是该字符串,若是则count加1。
3. 循环执行第2步,直到整个字符串被扫描完毕。
4. 输出count即为指定字符串出现的次数。
以下是具体的C语言代码实现:
```
#include <stdio.h>
#include <string.h>
int main()
{
char str[100] = "hello world, hello world!";
char substr[10] = "hello";
int count = 0;
char *p = str;
while(p = strchr(p, substr[0])) // 如果找到了第一个字符
{
if(strstr(p, substr) == p) // 如果匹配成功
{
count++;
}
p++; // 继续向后查找
}
printf("The number of substrings in the string is %d", count);
return 0;
}
```
相关问题
c++用vector输入字符串
在C++中,可以使用vector来输入字符串。以下是一个示例代码:
```cpp
#include <iostream>
#include <vector>
#include <string>
int main() {
std::vector<std::string> strings; // 创建存储字符串的vector
std::string input; // 创建一个字符串变量用于接收输入
while (std::cin >> input) {
strings.push_back(input); // 将输入的字符串添加到vector中
char c = std::cin.get(); // 检查输入的字符是否为换行符
if (c == '\n') {
break; // 如果是换行符,结束输入循环
}
}
for (const auto& str : strings) {
std::cout << str << " "; // 打印所有输入的字符串
}
return 0;
}
```
在上述代码中,我们使用了一个循环来不断读取输入的字符串并将其添加到vector中。当遇到换行符时,循环结束。然后,我们使用另一个循环来打印所有输入的字符串。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* *2* *3* [C++动态输入一个Vector或Vector当作输入接口](https://blog.csdn.net/wangzai32100/article/details/126533089)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 100%"]
[ .reference_list ]
c++ vector一维字符串数组
C++中的vector是一个动态数组容器,可以存储不同类型的数据,包括字符串。一维字符串数组可以通过vector<string>来实现。
下面是使用vector创建一维字符串数组的示例代码:
```cpp
#include <iostream>
#include <vector>
#include <string>
int main() {
std::vector<std::string> strArray; // 创建一个空的字符串数组
// 向数组中添加元素
strArray.push_back("Hello");
strArray.push_back("World");
strArray.push_back("C++");
// 遍历数组并输出每个字符串
for (const auto& str : strArray) {
std::cout << str << " ";
}
std::cout << std::endl;
return 0;
}
```
运行以上代码,输出结果为:
```
Hello World C++
```
相关推荐
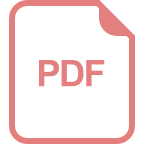
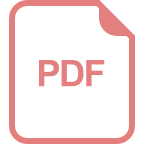












