#include<iostream> #include<cstring> using namespace std; int strlen(const char *str) { int i = 0; while (str[i] != '\0') { i++; } return i; } int main() { char s[] = "Hello world"; cout << strlen(s) << endl; system("pause"); return 0; }
时间: 2024-01-28 22:03:20 浏览: 29
这段代码实现了一个自定义的字符串长度函数 `strlen`,其功能与标准库中的 `strlen` 函数相同。具体来说,它接受一个指向字符数组的指针 `const char *str`,然后遍历该字符数组直到遇到了字符串的结束符 `\0`,最后返回字符串的长度。
在 `main` 函数中,我们定义了一个字符数组 `s` 并初始化为 `"Hello world"`。然后调用了自定义的 `strlen` 函数来计算字符串的长度,并将结果输出到屏幕上。
最后,通过调用系统函数 `system("pause")` 来暂停程序的执行,以便我们能够在控制台中看到程序的输出结果。
相关问题
#include<iostream> #include<cstring> using namespace std; int strlen(const char *str) { int i = 0; while (str[i] != '\0') { i++; } return i; } int main() { char s[] = "Hello world"; cout << strlen(s) << endl; system("pause"); return 0; }这段代码的问题
这段代码没有明显的语法错误,但是有一些可以优化的地方。
首先,自定义的 `strlen` 函数与标准库中的 `strlen` 函数重名,这可能会引起一些混淆和问题,因此最好将函数名改为不同的名称。
其次,程序中使用了 `system("pause")` 函数来暂停程序的执行,这种做法并不好,因为它会调用操作系统的命令行窗口来实现暂停,而且在某些平台上可能会出现问题。更好的做法是使用 `cin.get()` 函数来等待用户输入任意字符,以达到暂停程序的目的。
最后,由于程序中只是输出了字符串的长度,因此可以将 `char s[] = "Hello world";` 改为 `const char *s = "Hello world";`,这样可以避免不必要的内存分配和拷贝操作。
改进后的代码如下所示:
```cpp
#include <iostream>
#include <cstring>
using namespace std;
int my_strlen(const char* str)
{
int i = 0;
while (str[i] != '\0')
{
i++;
}
return i;
}
int main()
{
const char* s = "Hello world";
cout << my_strlen(s) << endl;
cin.get();
return 0;
}
```
这样修改后,代码更加简洁和可读,也更加安全和稳定。
#include <iostream> #include <cstdio> #include <cstring> using namespace std; class STRING{ char* pstr; public: STRING(const char* s="");//已实现 ~STRING();//已实现 friend istream& operator>>(istream& in, STRING& s); friend ostream& operator<<(ostream& os, const STRING& s); }; int main() { STRING s; cin >> s; cout << s << endl; return 0; } /* 请在这里填写答案,,你只需要实现输入输出运算符(>>、<<)的重载即可 */
可以这样实现输入输出运算符的重载:
```c++
#include <iostream>
#include <cstdio>
#include <cstring>
using namespace std;
class STRING{
char* pstr;
public:
STRING(const char* s="");
~STRING();
friend istream& operator>>(istream& in, STRING& s);
friend ostream& operator<<(ostream& os, const STRING& s);
};
STRING::STRING(const char* s) {
pstr = new char[strlen(s)+1];
strcpy(pstr, s);
}
STRING::~STRING() {
delete []pstr;
}
istream& operator>>(istream& in, STRING& s) {
char buffer[1024];
in.getline(buffer, 1024);
s.pstr = new char[strlen(buffer)+1];
strcpy(s.pstr, buffer);
return in;
}
ostream& operator<<(ostream& os, const STRING& s) {
os << s.pstr;
return os;
}
int main() {
STRING s;
cin >> s;
cout << s << endl;
return 0;
}
```
在输入运算符中,我们使用`getline()`函数来读取输入的字符串,然后动态分配空间并将字符串拷贝到对象的数据成员中。
在输出运算符中,我们直接输出对象的数据成员。
注意,在使用动态分配空间时,需要在析构函数中释放空间。
阅读全文
相关推荐
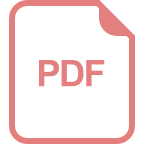
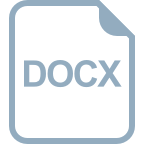
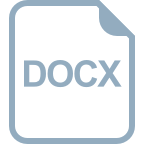













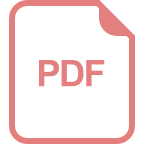