C++中,如何将std::vector<std::string> result这个数组清空
时间: 2024-01-19 18:04:38 浏览: 28
在C++中,可以使用以下两种方法清空 `std::vector<std::string>` 数组:
1. 使用`clear()`函数清空:
```c++
std::vector<std::string> result;
// 向 result 中添加一些元素
result.clear(); // 清空 result 数组
```
2. 使用`resize()`函数清空:
```c++
std::vector<std::string> result;
// 向 result 中添加一些元素
result.resize(0); // 清空 result 数组
```
无论使用哪种方法,都可以将 `std::vector<std::string>` 数组清空。建议使用 `clear()` 函数来清空 `std::vector` 数组,因为它更加简洁明了。
相关问题
std::cout能直接输出vector<std::string>吗
std::cout是C++标准库中的输出流对象,它可以用于输出各种类型的数据。然而,对于vector<std::string>类型的数据,std::cout不能直接输出整个容器。你需要使用循环遍历vector,并逐个输出其中的每个元素。
下面是一个示例代码,展示了如何使用std::cout输出vector<std::string>中的每个元素:
```cpp
#include <iostream>
#include <vector>
#include <string>
int main() {
std::vector<std::string> vec = {"Hello", "C++", "World"};
for (const auto& str : vec) {
std::cout << str << " ";
}
return 0;
}
```
上述代码中,我们使用了范围-based for循环来遍历vector中的每个元素,并使用std::cout输出每个元素。输出结果为:"Hello C++ World"。
std::vector<std::string>
std::vector<std::string>是C++标准库中的一个容器类,用于存储一组字符串。它提供了动态数组的功能,可以根据需要自动调整大小。
使用std::vector<std::string>可以方便地进行字符串的添加、删除、查找等操作。以下是std::vector<std::string>的一些常用操作:
1. 添加元素:可以使用push_back()函数将一个字符串添加到std::vector<std::string>的末尾。
2. 删除元素:可以使用erase()函数删除指定位置的字符串,或者使用pop_back()函数删除末尾的字符串。
3. 访问元素:可以使用下标运算符[]来访问指定位置的字符串,也可以使用at()函数来进行访问,at()函数会进行边界检查。
4. 获取大小:可以使用size()函数获取std::vector<std::string>中元素的个数。
5. 清空容器:可以使用clear()函数清空std::vector<std::string>中的所有元素。
6. 遍历容器:可以使用迭代器来遍历std::vector<std::string>中的所有元素。
相关推荐
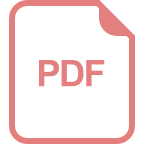
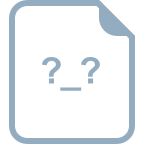
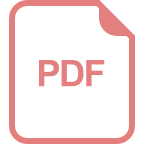












