win32 api绘制按钮,绘制文字。用户点击此按钮,在窗口中重绘其它新的文字
时间: 2023-06-25 22:08:22 浏览: 99
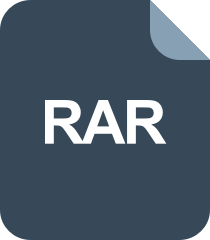
win32环境下的按钮重绘

要绘制按钮和文字,可以使用Win32 API中的CreateWindowEx和DrawText函数。CreateWindowEx用于创建按钮,DrawText用于绘制文本。
以下是一个简单的示例程序,可以创建一个按钮和一个静态文本框。当用户点击按钮时,程序会在文本框中显示新的文本。
```c++
#include <windows.h>
LRESULT CALLBACK WndProc(HWND hwnd, UINT msg, WPARAM wParam, LPARAM lParam);
int WINAPI WinMain(HINSTANCE hInstance, HINSTANCE hPrevInstance, LPSTR lpCmdLine, int nCmdShow)
{
WNDCLASSEX wc;
HWND hwnd;
MSG Msg;
// Step 1: Registering the Window Class
wc.cbSize = sizeof(WNDCLASSEX);
wc.style = 0;
wc.lpfnWndProc = WndProc;
wc.cbClsExtra = 0;
wc.cbWndExtra = 0;
wc.hInstance = hInstance;
wc.hIcon = LoadIcon(NULL, IDI_APPLICATION);
wc.hCursor = LoadCursor(NULL, IDC_ARROW);
wc.hbrBackground = (HBRUSH)(COLOR_WINDOW + 1);
wc.lpszMenuName = NULL;
wc.lpszClassName = "MyClass";
wc.hIconSm = LoadIcon(NULL, IDI_APPLICATION);
if (!RegisterClassEx(&wc))
{
MessageBox(NULL, "Window Registration Failed!", "Error!", MB_ICONEXCLAMATION | MB_OK);
return 0;
}
// Step 2: Creating the Window
hwnd = CreateWindowEx(
WS_EX_CLIENTEDGE,
"MyClass",
"The Title of My Window",
WS_OVERLAPPEDWINDOW,
CW_USEDEFAULT, CW_USEDEFAULT, 240, 120,
NULL, NULL, hInstance, NULL);
if (hwnd == NULL)
{
MessageBox(NULL, "Window Creation Failed!", "Error!", MB_ICONEXCLAMATION | MB_OK);
return 0;
}
// Step 3: Show the Window
ShowWindow(hwnd, nCmdShow);
UpdateWindow(hwnd);
// Step 4: The Message Loop
while (GetMessage(&Msg, NULL, 0, 0) > 0)
{
TranslateMessage(&Msg);
DispatchMessage(&Msg);
}
return Msg.wParam;
}
LRESULT CALLBACK WndProc(HWND hwnd, UINT msg, WPARAM wParam, LPARAM lParam)
{
switch (msg)
{
case WM_CREATE:
// Create a button
CreateWindow(
"BUTTON",
"Click me!",
WS_VISIBLE | WS_CHILD | BS_DEFPUSHBUTTON,
10, 10, 100, 30,
hwnd,
(HMENU)1,
NULL,
NULL);
// Create a static text box
CreateWindow(
"STATIC",
"Hello, World!",
WS_VISIBLE | WS_CHILD,
10, 50, 200, 30,
hwnd,
(HMENU)2,
NULL,
NULL);
break;
case WM_COMMAND:
if (LOWORD(wParam) == 1) // Button clicked
{
// Get the handle to the static text box
HWND hStatic = GetDlgItem(hwnd, 2);
// Change the text in the static text box
SetWindowText(hStatic, "Button clicked!");
}
break;
case WM_PAINT:
{
PAINTSTRUCT ps;
HDC hdc = BeginPaint(hwnd, &ps);
// TODO: Add any drawing code here...
EndPaint(hwnd, &ps);
}
break;
case WM_DESTROY:
PostQuitMessage(0);
break;
default:
return DefWindowProc(hwnd, msg, wParam, lParam);
}
return 0;
}
```
在上面的示例程序中,我们在WM_CREATE消息处理程序中创建了一个按钮和一个静态文本框。当用户点击按钮时,我们在WM_COMMAND消息处理程序中获取静态文本框的句柄,并使用SetWindowText函数更改其文本内容。
要重新绘制窗口中的文本,可以在WM_PAINT消息处理程序中使用DrawText函数。您可以在此处添加绘制文本的代码。在绘制完文本后,使用EndPaint函数结束绘制过程。
阅读全文
相关推荐
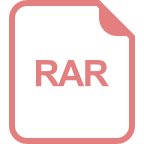
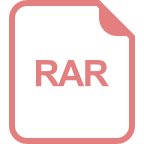
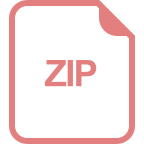
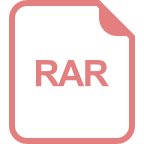
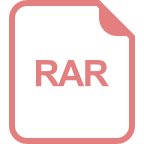
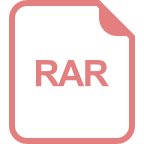
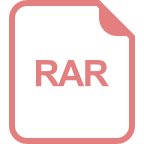
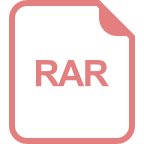
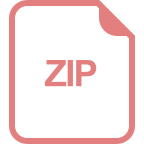
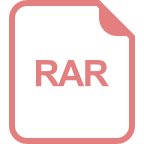
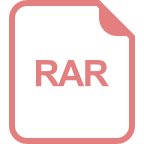
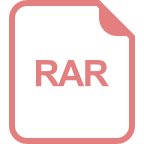
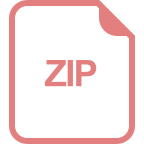
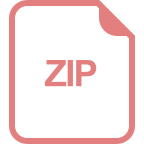