vue 简易在线客服功能
时间: 2023-10-15 18:06:21 浏览: 149
要在 Vue 中实现简易在线客服功能,你可以使用第三方库或者自己编写代码。
如果你想使用第三方库,可以考虑使用 `vue-chat-widget` 或 `vue-beautiful-chat`。这些库都提供了可定制的聊天窗口组件,你只需要将其添加到你的 Vue 应用中即可。
如果你想自己编写代码,可以按以下步骤操作:
1. 创建一个聊天窗口组件
你可以使用 Vue 的模板语法创建一个聊天窗口组件。该组件应该包含一个输入框和一个消息列表。
2. 处理用户输入
当用户在输入框中输入消息时,你需要将该消息添加到消息列表中。你可以使用 `v-model` 指令来绑定输入框的值,并在输入框上添加一个 `@keyup.enter` 监听器来处理用户按下回车键时的事件。
3. 处理客服回复
当客服回复消息时,你需要将该消息添加到消息列表中。你可以使用一个数组来存储消息,并在组件中循环遍历该数组以显示所有消息。
4. 实现在线客服功能
为了实现在线客服功能,你需要将用户的消息发送到服务器,并从服务器获取客服的回复。你可以使用 `axios` 库来发送网络请求。
当用户发送消息时,你可以将该消息作为参数发送到服务器,服务器将返回客服的回复。你可以在组件中添加一个方法来处理网络请求,并在该方法中更新消息列表。
当客服发送消息时,服务器可以使用 WebSockets 或长轮询等技术向客户端推送新消息。你可以使用 `socket.io` 库来实现 WebSockets 功能。
以上是实现简易在线客服功能的基本步骤,你可以根据自己的需求进行定制和扩展。
相关问题
vue2简易学生管理功能
Vue2是一个轻量级的前端JavaScript框架,用于构建用户界面。在开发一个简易的学生管理系统中,我们可以创建以下几个关键组件:
1. **学生列表** (Student List): 这个组件显示所有学生的列表,包含每个学生的基本信息如姓名、学号等,并提供搜索、排序等功能。
```html
<template>
<div>
<input v-model="searchTerm" placeholder="搜索学生...">
<table>
<tr>
<th>姓名</th>
<th>学号</th>
</tr>
<tr v-for="(student, index) in filteredStudents" :key="index">
<td>{{ student.name }}</td>
<td>{{ student.id }}</td>
</tr>
</table>
</div>
</template>
<script>
export default {
data() {
return {
searchTerm: "",
students: [], // 存储学生数据
};
},
computed: {
filteredStudents() {
return this.students.filter(student =>
student.name.toLowerCase().includes(this.searchTerm.toLowerCase())
);
}
},
};
</script>
```
2. **添加/编辑学生** (Add/Edit Student): 用户可以点击按钮新增学生,或者编辑已有学生的信息,通过表单输入姓名和学号等字段。
```html
<template>
<form @submit.prevent="saveStudent">
<input type="text" v-model="newStudent.name" placeholder="姓名">
<input type="text" v-model="newStudent.id" placeholder="学号">
<button type="submit">保存</button>
</form>
</template>
<script>
export default {
methods: {
saveStudent() {
// 实际应用中,这里会发送请求到服务器
this.students.push(this.newStudent);
this.newStudent = {}; // 清空表单
},
},
data() {
return {
newStudent: { name: "", id: "" },
};
},
};
</script>
```
3. **删除学生** (Delete Student): 提供一个确认删除的弹窗,当用户选择某个学生并确认删除操作时,从列表中移除该学生。
```html
<template>
<button @click="confirmDelete(student)">删除</button>
</template>
<script>
export default {
methods: {
confirmDelete(student) {
this.$confirm('确定要删除吗?', '警告', {
confirmButtonText: '删除',
cancelButtonText: '取消',
type: 'warning'
}).then(() => {
this.students.splice(this.students.indexOf(student), 1);
});
},
},
};
</script>
```
vue简易商品表格,含有修改功能
Vue.js 简易的商品表格通常会结合模板、数据绑定和事件处理机制来实现。首先,你需要创建一个包含商品信息的数据数组,然后在HTML模板中使用`v-for`指令遍历这个数组,显示每个商品的名称、价格等基本信息。对于修改功能,你可以设置每个单元格为可编辑状态,并添加点击事件,触发表单输入框的聚焦以及修改操作。
以下是一个简化的示例:
```html
<template>
<table>
<thead>
<tr>
<th>商品ID</th>
<th>商品名称</th>
<th>价格</th>
<th>操作</th>
</tr>
</thead>
<tbody>
<tr v-for="(product, index) in products" :key="index">
<td>{{ product.id }}</td>
<td contenteditable="true">{{ product.name }}</td>
<td contenteditable="true">{{ product.price }}</td>
<td><button @click="editProduct(index)">编辑</button></td>
</tr>
</tbody>
<tfoot>
<tr>
<td colspan="4">
<form @submit.prevent="updateProduct">
<input type="text" v-model="selectedProduct.id" placeholder="ID" />
<input type="text" v-model="selectedProduct.name" placeholder="名称" />
<input type="number" v-model="selectedProduct.price" placeholder="价格" />
<button type="submit">保存</button>
</form>
</td>
</tr>
</tfoot>
</table>
</template>
<script>
export default {
data() {
return {
products: [
{ id: 1, name: '商品A', price: 100 },
// 更多商品...
],
selectedProduct: null,
};
},
methods: {
editProduct(index) {
this.selectedProduct = this.products[index];
},
updateProduct() {
const updatedProduct = Object.assign({}, this.selectedProduct); // 拷贝一份原始数据
// 更新数据库或局部状态
this.products[this.products.findIndex(product => product.id === updatedProduct.id)] = updatedProduct;
this.selectedProduct = null; // 清空已选择的产品
},
},
};
</script>
```
在这个例子中,当用户点击“编辑”按钮,当前行的商品会被复制到表单中。用户在表单内修改内容后,`updateProduct`方法会被调用,更新数据库或本地存储。注意这里只是一个基础示例,实际应用中可能还需要考虑更多的边界情况和验证。
阅读全文
相关推荐
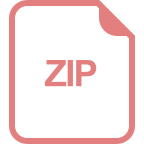
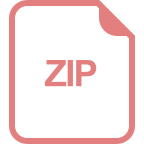
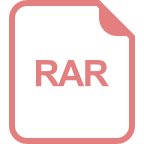













