用JAVA语言编写代码
时间: 2024-12-15 14:27:19 浏览: 2
您希望使用Java语言编写动态规划算法来解决矩阵链乘法问题和最长公共子序列(LCS)问题。以下是两个问题的Java实现示例:
### 矩阵链乘法问题
```java
public class MatrixChainMultiplication {
public static int matrixChainOrder(int[] p, int[][] m, int i, int j) {
if (i == j) return 0;
m[i][j] = Integer.MAX_VALUE;
for (int k = i; k < j; k++) {
int q = matrixChainOrder(p, m, i, k) + matrixChainOrder(p, m, k + 1, j) + p[i - 1] * p[k] * p[j];
if (q < m[i][j]) {
m[i][j] = q;
}
}
return m[i][j];
}
public static void main(String[] args) {
int[] p = {30, 2, 40, 10, 25};
int n = p.length;
int[][] m = new int[n][n];
System.out.println("Minimum number of multiplications is " + matrixChainOrder(p, m, 1, n - 1));
}
}
```
### 最长公共子序列(LCS)问题
```java
public class LongestCommonSubsequence {
public static String lcs(String X, String Y) {
int m = X.length();
int n = Y.length();
int[][] L = new int[m + 1][n + 1];
// Build the table in bottom-up fashion
for (int i = 0; i <= m; i++) {
for (int j = 0; j <= n; j++) {
if (i == 0 || j == 0)
L[i][j] = 0;
else if (X.charAt(i - 1) == Y.charAt(j - 1))
L[i][j] = L[i - 1][j - 1] + 1;
else
L[i][j] = Math.max(L[i - 1][j], L[i][j - 1]);
}
}
// Following code is used to print LCS
StringBuilder sb = new StringBuilder();
int index = L[m][n];
int temp = index;
char[] lcs = new char[index + 1];
lcs[index] = '\0'; // Set the terminating character
// Start from the right-most-bottom-most corner and one by one store characters in lcs[]
int i = m, j = n;
while (i > 0 && j > 0) {
// If current character in X[] and Y are same, then current character is part of LCS
if (X.charAt(i - 1) == Y.charAt(j - 1)) {
lcs[index - 1] = X.charAt(i - 1); // Put current character in result
i--;
j--;
index--; // reduce values of i, j and index
} else if (L[i - 1][j] > L[i][j - 1])
i--;
else
j--;
}
return new String(lcs);
}
public static void main(String[] args) {
String X = "GTTCCATA";
String Y = "CGATATTTGA";
System.out.println("LCS is " + lcs(X, Y));
}
}
```
这两个示例分别实现了矩阵链乘法问题和最长公共子序列问题的动态规划解决方案。您可以直接运行这些代码来验证结果。如果有任何其他问题或需要进一步的帮助,请告诉我!
阅读全文
相关推荐
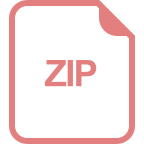
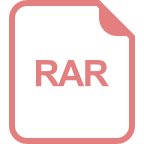
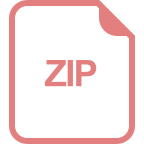















