2. 用C++某学校的图书管理系统中,书本种类信息包括:书号、书名、价格三项属性,且书的种类数不超过20。编写代码,分别用顺序存储方式和链式存储方式实现该图书管理系统数据结构的构建,并至少能够进行初始化、清空、增加、删除、查找操作。
时间: 2024-11-13 08:26:51 浏览: 23
在C++中,我们通常会使用数组或动态数组(vector)来表示顺序存储,而链表(如`std::list`或自定义节点的链表)来实现链式存储。
**顺序存储(数组)实现:**
```cpp
#include <iostream>
#include <string>
const int MAX_BOOKS = 20;
struct Book {
std::string bookID;
std::string title;
double price;
};
class Library {
private:
Book books[MAX_BOOKS];
int count = 0;
public:
// 初始化
void init() {
for (int i = 0; i < MAX_BOOKS; i++) {
books[i].bookID = "";
books[i].title = "";
books[i].price = 0.0;
}
}
// 清空
void clear() {
count = 0;
for (int i = 0; i < MAX_BOOKS; i++) {
books[i] = { "", "", 0.0 };
}
}
// 添加书籍
void addBook(const std::string& id, const std::string& title, double price) {
if (count < MAX_BOOKS) {
books[count] = { id, title, price };
count++;
} else {
std::cout << "图书馆已满,无法添加新书。\n";
}
}
// 删除书籍
bool removeBook(const std::string& id) {
for (int i = 0; i < count; i++) {
if (books[i].bookID == id) {
for (int j = i; j < count - 1; j++) {
books[j] = books[j + 1];
}
count--;
return true;
}
}
return false; // 没找到对应书籍
}
// 查找书籍
bool findBook(const std::string& id, Book& book) const {
for (int i = 0; i < count; i++) {
if (books[i].bookID == id) {
book = books[i];
return true;
}
}
return false;
}
};
// 使用示例
Library lib;
lib.init();
lib.addBook("001", "C++入门", 59.9);
lib.findBook("001", lib.books[0]); // 查找并打印结果
```
**链式存储(链表)实现:**
```cpp
#include <iostream>
#include <string>
struct BookNode {
std::string bookID;
std::string title;
double price;
BookNode* next;
};
class Node {
public:
explicit Node(std::string id, std::string title, double price) : bookID(id), title(title), price(price), next(nullptr) {}
};
class DynamicListLibrary {
private:
Node* head;
public:
// 初始化
void init() {
head = nullptr;
}
// 清空
void clear() {
Node* current = head;
while (current) {
Node* temp = current;
current = current->next;
delete temp;
}
head = nullptr;
}
// 添加书籍
void addBook(const std::string& id, const std::string& title, double price) {
Node* newNode = new Node(id, title, price);
if (!head) {
head = newNode;
} else {
newNode->next = head;
head = newNode;
}
}
// 删除书籍
bool removeBook(const std::string& id) {
Node* current = head;
while (current) {
if (current->bookID == id) {
Node* temp = current;
current = current->next;
delete temp;
if (!current) {
head = nullptr;
} else {
head = current;
}
return true;
}
}
return false;
}
// 查找书籍
bool findBook(const std::string& id, BookNode*& node) const {
Node* current = head;
while (current) {
if (current->bookID == id) {
node = current;
return true;
}
current = current->next;
}
node = nullptr;
return false;
}
};
// 使用示例
DynamicListLibrary dlLib;
dlLib.init();
dlLib.addBook("001", "C++入门", 59.9);
dlLib.findBook("001", dlLib.head); // 查找并打印结果
```
阅读全文
相关推荐
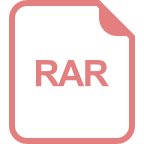
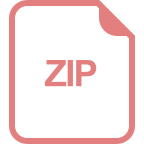
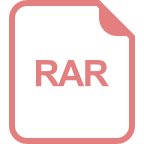






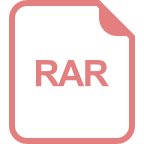
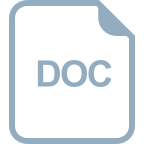
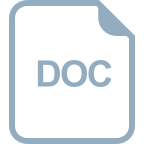

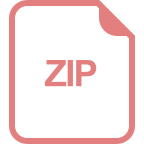
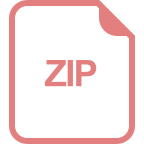
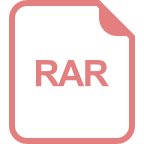
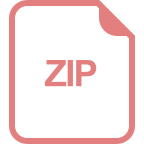
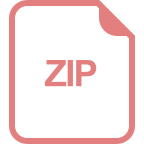