HTML5,JS,VUE.js网页游戏的附录
时间: 2023-08-26 17:03:33 浏览: 44
以下是 HTML5、JS 和 Vue.js 网页游戏开发时可能用到的一些附录:
## HTML5
### HTML5 标准游戏循环
```
var lastTime = 0;
var vendors = ['ms', 'moz', 'webkit', 'o'];
for(var x = 0; x < vendors.length && !window.requestAnimationFrame; ++x) {
window.requestAnimationFrame = window[vendors[x]+'RequestAnimationFrame'];
window.cancelAnimationFrame = window[vendors[x]+'CancelAnimationFrame'] || window[vendors[x]+'CancelRequestAnimationFrame'];
}
if (!window.requestAnimationFrame)
window.requestAnimationFrame = function(callback, element) {
var currTime = new Date().getTime();
var timeToCall = Math.max(0, 16 - (currTime - lastTime));
var id = window.setTimeout(function() { callback(currTime + timeToCall); },
timeToCall);
lastTime = currTime + timeToCall;
return id;
};
if (!window.cancelAnimationFrame)
window.cancelAnimationFrame = function(id) {
clearTimeout(id);
};
```
### HTML5 音频开关控制
```
var soundOn = true;
function toggleSound() {
soundOn = !soundOn;
}
```
## JavaScript
### JS 获取 DOM 元素
```
var canvas = document.getElementById("canvas");
var ctx = canvas.getContext("2d");
```
### JS 数组随机排序
```
function shuffle(array) {
var currentIndex = array.length, temporaryValue, randomIndex;
// While there remain elements to shuffle...
while (0 !== currentIndex) {
// Pick a remaining element...
randomIndex = Math.floor(Math.random() * currentIndex);
currentIndex -= 1;
// And swap it with the current element.
temporaryValue = array[currentIndex];
array[currentIndex] = array[randomIndex];
array[randomIndex] = temporaryValue;
}
return array;
}
```
### JS 生成随机数
```
function getRandomInt(min, max) {
return Math.floor(Math.random() * (max - min + 1)) + min;
}
```
### JS 碰撞检测
```
function collisionDetection(obj1, obj2) {
if (obj1.x < obj2.x + obj2.width &&
obj1.x + obj1.width > obj2.x &&
obj1.y < obj2.y + obj2.height &&
obj1.y + obj1.height > obj2.y) {
return true;
}
return false;
}
```
## Vue.js
### Vue.js 组件注册
```
Vue.component('my-component', {
template: '<div>A custom component!</div>'
})
```
### Vue.js 组件通信
```
// 父组件
<template>
<div>
<child-component v-on:custom-event="handleCustomEvent"></child-component>
</div>
</template>
<script>
import ChildComponent from './ChildComponent.vue';
export default {
components: {
ChildComponent
},
methods: {
handleCustomEvent(data) {
console.log(data);
}
}
};
</script>
// 子组件
<template>
<div>
<button v-on:click="emitCustomEvent">Click me!</button>
</div>
</template>
<script>
export default {
methods: {
emitCustomEvent() {
this.$emit('custom-event', 'Custom event data!');
}
}
};
</script>
```
相关推荐
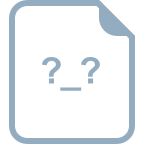
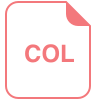
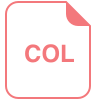
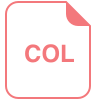
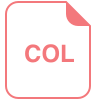
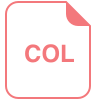









