Cannot deserialize value of type `java.time.LocalDateTime` from String "2023-05-22 17:11:44":
时间: 2023-09-23 09:03:34 浏览: 123
这个错误通常是因为你在使用Jackson或其他JSON库将一个字符串转换为`LocalDateTime`对象时,格式不匹配。正确的格式应该是`yyyy-MM-dd HH:mm:ss`,而你提供的字符串格式为`yyyy-MM-dd HH:mm:ss`,缺少了秒的部分。
你可以尝试使用`DateTimeFormatter`来解析这个字符串,例如:
```
String dateString = "2023-05-22 17:11:44";
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
LocalDateTime dateTime = LocalDateTime.parse(dateString, formatter);
```
这将使用指定的格式解析日期字符串,并返回一个`LocalDateTime`对象。
相关问题
2023-05-24 16:39:43.677 WARN 23684 --- [nio-8080-exec-1] .w.s.m.s.DefaultHandlerExceptionResolver : Resolved [org.springframework.http.converter.HttpMessageNotReadableException: JSON parse error: Cannot deserialize value of type `java.time.LocalDateTime` from String "2023-05-24 00:00:00": Failed to deserialize java.time.LocalDateTime: (java.time.format.DateTimeParseException) Text '2023-05-24 00:00:00' could not be parsed at index 10; nested exception is com.fasterxml.jackson.databind.exc.InvalidFormatException: Cannot deserialize value of type `java.time.LocalDateTime` from String "2023-05-24 00:00:00": Failed to deserialize java.time.LocalDateTime: (java.time.format.DateTimeParseException) Text '2023-05-24 00:00:00' could not be parsed at index 10<EOL> at [Source: (org.springframework.util.StreamUtils$NonClosingInputStream); line: 12, column: 22] (through reference chain: cc.wellcloud.cloud.dam3.model.entity.PlanEntity["backupBeginTime"])]
这个错误信息是由于在反序列化JSON字符串时,无法将字符串“2023-05-24 00:00:00”转换为LocalDateTime类型。DateTimeParseException 表示时间解析异常,它指示日期时间格式不符合ISO-8601格式。你可以检查一下传递给反序列化方法的日期时间格式是否正确。如果日期时间格式正确,那么你可以尝试使用指定的格式来反序列化日期时间字符串。例如,如果日期时间字符串的格式为“yyyy-MM-dd HH:mm:ss”,你可以使用以下代码将其转换为 LocalDateTime 类型:
```java
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
LocalDateTime dateTime = LocalDateTime.parse("2023-05-24 00:00:00", formatter);
```
这样就可以将字符串转换为 LocalDateTime 类型了。
Cannot deserialize value of type `java.time.LocalDateTime` from String "2023-05-22 17:11:44" 全局配置解决方案
这个问题是因为在反序列化的过程中,无法将字符串 "2023-05-22 17:11:44" 转换为 LocalDateTime 类型。解决方案可以在全局配置文件中配置一个 LocalDateTime 的序列化和反序列化器。
首先,创建一个 LocalDateTime 序列化器:
```java
import java.io.IOException;
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
import com.fasterxml.jackson.core.JsonGenerator;
import com.fasterxml.jackson.databind.JsonSerializer;
import com.fasterxml.jackson.databind.SerializerProvider;
public class LocalDateTimeSerializer extends JsonSerializer<LocalDateTime> {
private static final DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
@Override
public void serialize(LocalDateTime value, JsonGenerator gen, SerializerProvider serializers) throws IOException {
gen.writeString(formatter.format(value));
}
}
```
然后,创建一个 LocalDateTime 反序列化器:
```java
import java.io.IOException;
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
import com.fasterxml.jackson.core.JsonParser;
import com.fasterxml.jackson.databind.DeserializationContext;
import com.fasterxml.jackson.databind.JsonDeserializer;
public class LocalDateTimeDeserializer extends JsonDeserializer<LocalDateTime> {
private static final DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss");
@Override
public LocalDateTime deserialize(JsonParser p, DeserializationContext ctxt) throws IOException {
String str = p.getText().trim();
return LocalDateTime.parse(str, formatter);
}
}
```
最后,在全局配置文件中注册这两个序列化器和反序列化器:
```yaml
spring:
jackson:
date-format: yyyy-MM-dd HH:mm:ss
serialization:
write-dates-as-timestamps: false
modules:
- com.fasterxml.jackson.datatype.jsr310.JavaTimeModule
property-naming-strategy: SNAKE_CASE
deserialization:
local-date-time:
date-time-formatter: "yyyy-MM-dd HH:mm:ss"
type: java.time.LocalDateTime
key-deserializer: com.example.LocalDateTimeDeserializer
value-deserializer: com.example.LocalDateTimeDeserializer
creators:
use-big-decimal-for-floats: true
```
这样就可以在反序列化时将字符串转换为 LocalDateTime 类型了。
阅读全文
相关推荐
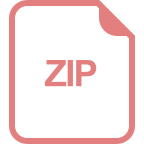
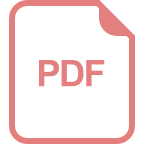
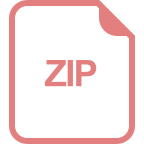














