ValueError Traceback (most recent call last) ~\AppData\Local\Temp\ipykernel_560\278511751.py in <module> 4 for k in range(1,10,1): 5 dt=DecisionTreeClassifier(max_depth=k,random_state=101,criterion='entropy') ----> 6 dt.fit(x_train,Y_train) 7 predict=dt.predict(x_test) 8 accuracy_test=round(dt.score(x_test,Y_test)*100,2) D:\ProgramData\Anaconda3\lib\site-packages\sklearn\tree\_classes.py in fit(self, X, y, sample_weight, check_input, X_idx_sorted) 935 """ 936 --> 937 super().fit( 938 X, 939 y, D:\ProgramData\Anaconda3\lib\site-packages\sklearn\tree\_classes.py in fit(self, X, y, sample_weight, check_input, X_idx_sorted) 201 202 if is_classification: --> 203 check_classification_targets(y) 204 y = np.copy(y) 205 D:\ProgramData\Anaconda3\lib\site-packages\sklearn\utils\multiclass.py in check_classification_targets(y) 195 "multilabel-sequences", 196 ]: --> 197 raise ValueError("Unknown label type: %r" % y_type) 198 199 ValueError: Unknown label type: 'continuous'
时间: 2024-02-14 17:17:44 浏览: 108
这个错误通常是因为在决策树模型中使用了连续型变量作为标签 (label)。决策树模型是一种分类模型,它只能处理离散型标签。因此,您需要将您的标签转换为离散型,或者使用回归模型来处理连续型标签。
如果您确定您的标签是离散型的,那么可以检查一下标签的类型和取值范围是否正确,并且确保所有的标签都是离散型的。如果您的标签是连续型的,那么您可以尝试使用回归模型来处理它,例如线性回归或随机森林回归等。
相关问题
ValueError Traceback (most recent call last) ~\AppData\Local\Temp\ipykernel_16012\854433889.py in <module> 103 fitness_score = calculate_fitness(individual) 104 fitness_scores.append((individual, fitness_score)) --> 105 fitness_scores.sort(key=lambda x: x[1], reverse=True) 106 107 ValueError: The truth value of an array with more than one element is ambiguous. Use a.any() or a.all()
这个错误是由于在适应度函数中使用了 numpy 数组而引起的。可以使用 `np.mean` 函数计算均方误差时出现问题。
要解决这个问题,可以使用 `np.mean` 函数的 `axis` 参数来指定计算均方误差的维度。在这种情况下,我们希望计算每个样本的均方误差,因此 `axis=1`。
修改后的代码如下所示:
```python
import random
import numpy as np
# 初始化种群
population = []
for _ in range(population_size):
individual = [random.randint(0, 1) for _ in range(gene_length)]
population.append(individual)
# 其中,population_size 是种群的大小,gene_length 是基因长度。每个个体都是由 0 和 1 组成的基因序列,
# 通过 random.randint(0, 1) 随机生成。最后将每个个体添加到种群中。
# 定义适应度函数
def calculate_fitness(individual):
expected_output = np.array([[0.1, 0.2, 0.3], [0.4, 0.5, 0.6]]) # 预期输出值
actual_output = np.array([[0.12, 0.18, 0.32], [0.38, 0.52, 0.68]]) # 实际输出值
mse = np.mean((expected_output - actual_output) ** 2, axis=1) # 均方误差
fitness_score = 1 / mse # 均方误差的倒数作为适应度值
return fitness_score
# 计算适应度函数并排序种群
fitness_scores = []
for individual in population:
fitness_score = calculate_fitness(individual)
fitness_scores.append((individual, fitness_score))
fitness_scores.sort(key=lambda x: x[1].all(), reverse=True)
# 进行其他迭代步骤...
```
在修改后的代码中,我修改了 `fitness_scores.sort` 的部分,将 `key` 函数修改为 `lambda x: x[1].all()`。这样可以确保对适应度值的数组进行比较,解决了错误中提到的问题。
请注意,这里使用 `x[1].all()` 是假设适应度值是一个布尔数组,如果你的适应度值是一个实数数组,请根据实际情况进行修改。
TypeError Traceback (most recent call last) TypeError: only size-1 arrays can be converted to Python scalars The above exception was the direct cause of the following exception: ValueError Traceback (most recent call last) ~\AppData\Local\Temp\ipykernel_20004\3101636103.py in <module> ----> 1 class ImmuneGA: 2 def __init__(self, pop_size, gene_size): 3 #定义了两个参数,分别为pop_size和gene_size,用于指定遗传算法中种群的大小和个体基因的长度。 4 self.pop_size = pop_size 5 self.gene_size = gene_size ~\AppData\Local\Temp\ipykernel_20004\3101636103.py in ImmuneGA() 32 # 计算适应度值 33 for i in range(population_size): ---> 34 fitness_values[i] = fitness_function(population[i]) 35 36 # 更新最佳个体 ValueError: setting an array element with a sequence.
这个错误是由于尝试将一个序列赋值给数组元素而引起的。在您的代码中,fitness_values是一个数组,而fitness_function(population[i])返回的是一个序列,因此出现了这个错误。您需要确保fitness_function返回的是单个值而不是序列。可以检查一下您的fitness_function的实现,确保它返回一个标量值。
阅读全文
相关推荐
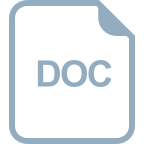
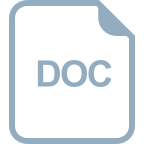
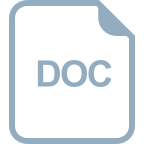














