ct107s开发板的ds1302读写函数
时间: 2023-06-25 18:03:05 浏览: 52
下面是ct107s开发板的ds1302读写函数的示例代码:
```c
#include "ct107s.h"
#include "ds1302.h"
// DS1302 RST引脚
#define DS1302_RST_PIN P0_0
// DS1302 IO引脚
#define DS1302_IO_PIN P0_1
// DS1302 SCLK引脚
#define DS1302_SCLK_PIN P0_2
// DS1302读取一个字节
u8 DS1302_ReadByte(void)
{
u8 i, dat = 0;
// 设置IO口为输入
GPIO_Init(DS1302_IO_PIN, GPIO_Mode_IPU);
// 读取数据
for (i = 0; i < 8; i++)
{
dat >>= 1;
GPIO_WriteBit(DS1302_SCLK_PIN, Bit_RESET);
if (GPIO_ReadInputDataBit(DS1302_IO_PIN) == Bit_SET)
{
dat |= 0x80;
}
GPIO_WriteBit(DS1302_SCLK_PIN, Bit_SET);
}
return dat;
}
// DS1302写入一个字节
void DS1302_WriteByte(u8 dat)
{
u8 i;
// 设置IO口为输出
GPIO_Init(DS1302_IO_PIN, GPIO_Mode_Out_PP);
// 写入数据
for (i = 0; i < 8; i++)
{
GPIO_WriteBit(DS1302_IO_PIN, (dat & 0x01) ? Bit_SET : Bit_RESET);
dat >>= 1;
GPIO_WriteBit(DS1302_SCLK_PIN, Bit_RESET);
GPIO_WriteBit(DS1302_SCLK_PIN, Bit_SET);
}
}
// DS1302读取一个寄存器
u8 DS1302_ReadReg(u8 reg)
{
u8 dat;
// 禁止写入
DS1302_WriteByte((reg << 1) | 0x81);
dat = DS1302_ReadByte();
// 使能写入
DS1302_WriteByte((reg << 1) | 0x80);
return dat;
}
// DS1302写入一个寄存器
void DS1302_WriteReg(u8 reg, u8 dat)
{
// 禁止写入
DS1302_WriteByte((reg << 1) | 0x80);
DS1302_WriteByte(dat);
// 使能写入
DS1302_WriteByte((reg << 1) | 0x81);
}
```
在使用时,需要先初始化IO口:
```c
GPIO_Init(DS1302_RST_PIN, GPIO_Mode_Out_PP);
GPIO_Init(DS1302_IO_PIN, GPIO_Mode_Out_PP);
GPIO_Init(DS1302_SCLK_PIN, GPIO_Mode_Out_PP);
```
然后就可以调用上述函数进行DS1302的读写操作了。例如,读取DS1302的秒数:
```c
u8 sec = DS1302_ReadReg(DS1302_SEC_REG);
```
写入DS1302的小时数:
```c
DS1302_WriteReg(DS1302_HOUR_REG, 0x12);
```
相关推荐
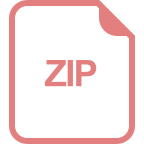
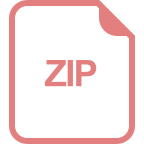
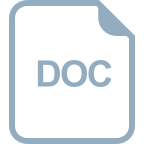
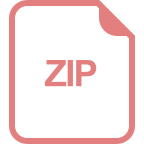
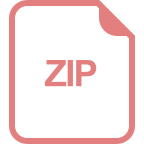
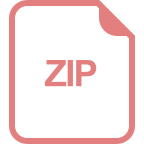
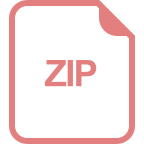
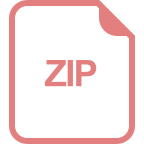
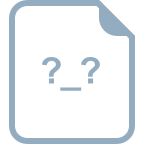
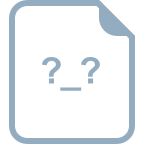