#include <stdio.h> #define MAXSIZE 100 int BinarySearch(int a[], int n, int k) { int low = 0, high = n - 1, mid; while (low <= high) { mid = (low + high) / 2; if (k == a[mid]) { return mid; } else if (k < a[mid]) { high = mid - 1; } else { low = mid + 1; } } return -1; } int main() { int a[MAXSIZE], n, k, i, pos; printf("请输入有序序列的长度n:"); scanf_s("%d", &n); printf("请输入有序序列:"); for (i = 0; i < n; i++) { scanf_s("%d", &a[i]); } printf("请输入要查找的关键字k:"); scanf_s("%d", &k); pos = BinarySearch(a, n, k); if (pos == -1) { printf("没有找到"); } else { printf("查找成功,下标为%d", pos); } return 0; } 根据代码画出完整的文字版流程图
时间: 2023-12-20 09:04:38 浏览: 15
开始
输入有序序列的长度n
输入n个元素到数组a中
输入要查找的关键字k
定义函数BinarySearch,传入参数a[],n,k
定义变量low=0,high=n-1,mid
当low<=high时,执行以下循环体
mid=(low+high)/2
如果k=a[mid],返回mid
如果k<a[mid],high=mid-1
否则,low=mid+1
返回-1
调用函数BinarySearch,传入参数a[],n,k,将返回值赋给pos
如果pos等于-1,输出没有找到
否则,输出查找成功,下标为pos
结束
相关问题
R6-3 二分查找 分数 17 作者 陈越 单位 浙江大学 本题要求实现二分查找算法。 函数接口定义: Position BinarySearch( List L, ElementType X ); 其中List结构定义如下: typedef int Position; typedef struct LNode *List; struct LNode { ElementType Data[MAXSIZE]; Position Last; /* 保存线性表中最后一个元素的位置 */ }; L是用户传入的一个线性表,其中ElementType元素可以通过>、==、<进行比较,并且题目保证传入的数据是递增有序的。函数BinarySearch要查找X在Data中的位置,即数组下标(注意:元素从下标1开始存储)。找到则返回下标,否则返回一个特殊的失败标记NotFound。 裁判测试程序样例: #include <stdio.h> #include <stdlib.h> #define MAXSIZE 10 #define NotFound 0 typedef int ElementType; typedef int Position; typedef struct LNode *List; struct LNode { ElementType Data[MAXSIZE]; Position Last; /* 保存线性表中最后一个元素的位置 */ }; List ReadInput(); /* 裁判实现,细节不表。元素从下标1开始存储 */ Position BinarySearch( List L, ElementType X ); int main() { List L; ElementType X; Position P; L = ReadInput(); scanf("%d", &X); P = BinarySearch( L, X ); printf("%d\n", P); return 0; } /* 你的代码将被嵌在这里 */ 输入样例1: 5 12 31 55 89 101 31 输出样例1: 2 输入样例2: 3 26 78 233 31 输出样例2: 0
下面是二分查找的实现代码:
```
Position BinarySearch(List L, ElementType X)
{
Position left = 1; // 左边界
Position right = L->Last; // 右边界
while (left <= right) {
Position mid = (left + right) / 2; // 中间位置
if (L->Data[mid] == X) { // 找到了
return mid;
} else if (L->Data[mid] > X) { // 往左边找
right = mid - 1;
} else { // 往右边找
left = mid + 1;
}
}
// 没找到
return NotFound;
}
```
具体思路是:首先设置左边界为1,右边界为L的最后一个元素的位置;然后在每一次循环中,取左右边界的平均值作为中间位置mid,并将L->Data[mid]和X进行比较,如果相等,则找到了,返回mid;如果L->Data[mid]比X大,则说明要在mid的左边继续查找,此时将右边界right更新为mid-1;否则说明要在mid的右边继续查找,此时将左边界left更新为mid+1。如果最终没有找到,返回NotFound。
本题要求实现两个函数:(1)排序;(2)二分查找 先利用排序算法将数据按关键字从小到大排序,再对排序后的数据进行二分查找 函数接口定义: void Sort(List L); //对用户传入的线性表进行排序 int BinarySearch( List L,int X ); //查找X,如果查找成功返回对应的数组下标位置;查找失败,则返回NotFound 其中List结构定义如下: struct LNode{ int Data[MAXSIZE]; //Data为待排序序列数组 int Last; //Last为最后一个元素的数组下标 }; typedef struct LNode *List; 裁判测试程序样例: #include <stdio.h> #include <stdlib.h> #define MAXSIZE 10 #define NotFound -1 struct LNode{ int Data[MAXSIZE]; //Data为待排序序列数组 int Last; //Last为最后一个元素的数组下标 }; typedef struct LNode *List; void Sort(List L); int BinarySearch( List L,int X ); List Create() { List L; L = (List)malloc(sizeof(struct LNode)); int j = 0,n,ch; scanf("%d",&n); for(j=0;j<n;j++) { scanf("%d",&ch); L->Data[j] = ch; } L->Last = n-1; return L; } int main() { List L; int x,p; L = Create(); scanf("%d", &x); //输入要查找的关键字 Sort(L); p = BinarySearch(L,x); if(p != -1){ printf("所查找数据的位置为:%d",p); }else{ printf("NotFound"); } return 0; } /* 请在这里填写答案 */ 输入样例1: 8 99 66 45 33 37 10 22 13 20 输出样例1: NotFound 输入样例2: 8 99 66 45 33 37 10 22 13 13 输出样例2: 所查找数据的位置为:1
```
void Sort(List L) {
int i, j, temp;
for (i = 0; i < L->Last; i++) {
for (j = i + 1; j <= L->Last; j++) {
if (L->Data[i] > L->Data[j]) {
temp = L->Data[i];
L->Data[i] = L->Data[j];
L->Data[j] = temp;
}
}
}
}
int BinarySearch(List L, int X) {
int left = 0, right = L->Last, mid;
while (left <= right) {
mid = (left + right) / 2;
if (L->Data[mid] == X) {
return mid;
} else if (L->Data[mid] > X) {
right = mid - 1;
} else {
left = mid + 1;
}
}
return NotFound;
}
```
相关推荐
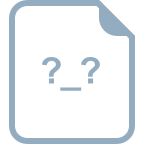
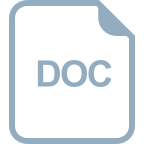
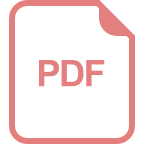
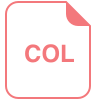












