python爬取b站评分
时间: 2023-08-21 13:04:59 浏览: 58
你可以使用Python来爬取B站视频的评分信息。以下是一个简单的示例代码,使用了requests和BeautifulSoup库来发送HTTP请求和解析HTML页面:
```python
import requests
from bs4 import BeautifulSoup
# 定义要爬取的视频链接
url = 'https://www.bilibili.com/video/av12345678'
# 发送HTTP请求获取页面内容
response = requests.get(url)
# 解析HTML页面
soup = BeautifulSoup(response.text, 'html.parser')
# 查找评分元素
rating_element = soup.find('span', class_='score')
# 获取评分值
rating = rating_element.text
print('视频评分:', rating)
```
请注意,你需要将代码中的`url`替换为你要爬取评分的B站视频链接。另外,这只是一个简单的示例,实际爬取过程可能会涉及到更多的处理和异常处理。此外,尽量遵守网站的爬虫规则,不要过度请求或对服务器造成负担。
相关问题
python爬取大众点评美食
你可以使用Python编写爬虫程序来爬取大众点评上的美食数据。以下是一个简单的示例程序:
```python
import requests
from bs4 import BeautifulSoup
def crawl_dianping():
url = "https://www.dianping.com/search/category/2/10"
headers = {
"User-Agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/88.0.4324.146 Safari/537.36"
}
response = requests.get(url, headers=headers)
soup = BeautifulSoup(response.text, "html.parser")
restaurants = soup.find_all("div", class_="txt")
for restaurant in restaurants:
name = restaurant.find("h4").text.strip()
score = restaurant.find("span", class_="comment-list").find("b").text.strip()
print("店名:", name)
print("评分:", score)
print("--------------------")
crawl_dianping()
```
这个程序使用了`requests`库发送HTTP请求,并使用`BeautifulSoup`库解析HTML页面。程序中的`url`是大众点评美食分类的URL,你可以根据需要修改URL来爬取其他分类的数据。
注意:在实际使用爬虫时,请遵守网站的爬虫规则,并合法、合理地使用爬虫技术。
python爬取网页电影信息
A:
要用Python爬取网页电影信息,你需要用到以下步骤:
1. 寻找目标网站:首先选择一个你要爬取的电影信息网站,确定网站的域名和访问方式。
2. 分析网站结构:查看你选择的网站的网页源代码,了解该网站的结构和元素,例如网页布局、CSS样式、JavaScript脚本等。
3. 安装Python库:使用Python进行网页爬取需要先安装一些Python库,如requests、beautifulsoup。
4. 使用 requests 库获取网页源代码:使用 requests 库向目标网站发送一个请求,然后获取网页的源代码。
5. 使用 beautifulsoup 库解析网页:使用 beautifulsoup 库来解析网页的 HTML 元素。
6. 提取电影信息:根据网页结构的特点,找到包含电影信息的 HTML 元素并提取它们。
7. 存储数据:最后一步是将提取到的数据存储在文件中,例如文本文件、CSV文件、JSON文件等。
下面是一个Python爬取电影信息的简单例子:
```python
import requests
from bs4 import BeautifulSoup
#请求目标网站资源
url = 'https://www.imdb.com/chart/top/?ref_=nv_mv_250'
response = requests.get(url)
soup = BeautifulSoup(response.text, 'html.parser')
#解析目标网站资源
movies = soup.select('td.titleColumn')
crew = [a.attrs.get('title') for a in soup.select('td.titleColumn a')]
ratings = [b.attrs.get('data-value') for b in soup.select('td.posterColumn span[name="ir"]')]
result = []
for idx in range(0, len(movies)):
title = movies[idx].text.strip()
year = title[-5:-1]
title = title[:-7]
place = movies[idx].span['data-value']
link = 'https://www.imdb.com/' + movies[idx].a['href']
rating = ratings[idx]
director_and_stars = crew[idx].split(',')
crew_director = director_and_stars[0].strip()
crew_stars = [star.strip() for star in director_and_stars[1:]]
result.append((place, title, year, rating, crew_director, crew_stars, link))
#显示数据
for movie in result:
print(movie)
```
该脚本通过 BeautifulSoup 库和 requests 库实现了获取和解析电影信息网站 IMDB 的 top250 网页数据。运行该脚本,你将会得到 IMDB top250 网页里所有电影的排名、电影名、上映年份、导演和演员、电影评分和电影海报链接等信息。
相关推荐
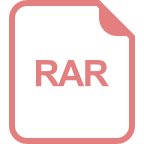
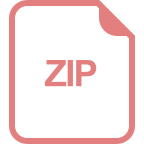












