使用列表、python内置函数完成成绩管理功能,要求统计人数、计算均分、最高分、最低分
时间: 2024-10-21 16:12:53 浏览: 22
在Python中,你可以使用列表来存储学生的成绩,并利用内置函数来实现成绩管理的功能。首先,创建一个包含学生成绩的列表示例:
```python
scores = [85, 90, 95, 78, 88, 92, 86] # 示例成绩列表
```
接下来,我们可以编写一些函数来完成所需的操作:
1. 统计人数(学生总数):
```python
def count_students(scores):
return len(scores)
total_students = count_students(scores)
print(f"总人数: {total_students}")
```
2. 计算均分(平均成绩):
```python
def calculate_average(scores):
return sum(scores) / len(scores)
average_score = calculate_average(scores)
print(f"平均分: {average_score}")
```
3. 找出最高分:
```python
def find_max_score(scores):
return max(scores)
highest_score = find_max_score(scores)
print(f"最高分: {highest_score}")
```
4. 找出最低分:
```python
def find_min_score(scores):
return min(scores)
lowest_score = find_min_score(scores)
print(f"最低分: {lowest_score}")
```
将以上代码组合在一起:
```python
scores = [85, 90, 95, 78, 88, 92, 86]
# 统计人数
total_students = count_students(scores)
# 计算平均分
average_score = calculate_average(scores)
# 找出最高分
highest_score = find_max_score(scores)
# 找出最低分
lowest_score = find_min_score(scores)
print(f"总人数: {total_students}")
print(f"平均分: {average_score}")
print(f"最高分: {highest_score}")
print(f"最低分: {lowest_score}")
```
运行此段代码会得到每个操作的结果。
阅读全文
相关推荐
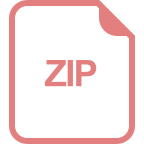
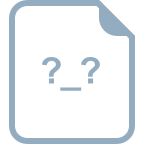
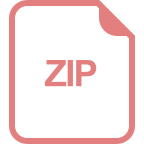
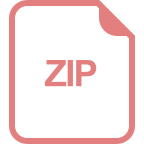
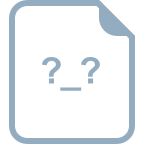
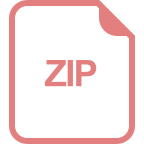
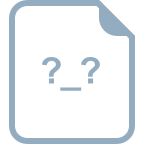
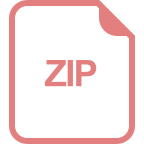
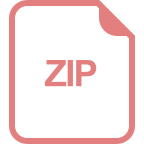