计算两点距离。成员函数 友元函数。全局函数
时间: 2023-09-15 12:20:06 浏览: 62
全局函数:
```c++
#include <cmath>
double distance(double x1, double y1, double x2, double y2) {
return sqrt(pow(x1 - x2, 2) + pow(y1 - y2, 2));
}
```
成员函数:
```c++
#include <cmath>
class Point {
public:
Point(double x, double y) : x_(x), y_(y) {}
double distance(Point other) {
return sqrt(pow(x_ - other.x_, 2) + pow(y_ - other.y_, 2));
}
private:
double x_;
double y_;
};
```
友元函数:
```c++
#include <cmath>
class Point {
public:
Point(double x, double y) : x_(x), y_(y) {}
friend double distance(Point p1, Point p2) {
return sqrt(pow(p1.x_ - p2.x_, 2) + pow(p1.y_ - p2.y_, 2));
}
private:
double x_;
double y_;
};
```
相关问题
计算两点之间距离(友元函数)
以下是一个计算两点之间距离的友元函数的示例代码:
```c++
#include <iostream>
#include <cmath>
using namespace std;
class Point {
private:
double x;
double y;
public:
Point(double x = 0, double y = 0) {
this->x = x;
this->y = y;
}
friend double distance(const Point& p1, const Point& p2);
};
double distance(const Point& p1, const Point& p2) {
double dx = p1.x - p2.x;
double dy = p1.y - p2.y;
return sqrt(dx * dx + dy * dy);
}
int main() {
Point p1(1, 2);
Point p2(4, 6);
double dist = distance(p1, p2);
cout << "The distance between point p1 and point p2 is " << dist << endl;
return 0;
}
```
在上述代码中,我们定义了一个 `Point` 类,并在其中声明了一个友元函数 `distance`。这个函数接受两个 `Point` 类型的参数,并计算它们之间的距离。
在 `main` 函数中,我们创建了两个 `Point` 对象,并调用了 `distance` 函数来计算它们之间的距离。最后,我们将结果输出到控制台上。
6-6 计算两点之间距离(友元函数)
以下是一个示例程序,演示如何通过友元函数计算两点之间的距离:
```c++
#include <iostream>
#include <cmath>
using namespace std;
class Point {
private:
double x;
double y;
public:
Point(double x = 0, double y = 0) : x(x), y(y) {}
friend double distance(const Point& p1, const Point& p2);
};
double distance(const Point& p1, const Point& p2) {
double dx = p1.x - p2.x;
double dy = p1.y - p2.y;
return sqrt(dx * dx + dy * dy);
}
int main() {
Point p1(1, 2);
Point p2(3, 4);
cout << "Distance between p1 and p2 is " << distance(p1, p2) << endl;
return 0;
}
```
在这个示例程序中,我们定义了一个 `Point` 类,该类具有两个私有成员变量 `x` 和 `y`,表示点的坐标。我们还定义了一个友元函数 `distance`,该函数接受两个 `Point` 类型的参数,并计算这两个点之间的距离。在 `Point` 类定义中,我们使用 `friend` 关键字将 `distance` 函数声明为友元函数,以便它可以访问 `Point` 类的私有成员变量。在 `main` 函数中,我们创建两个 `Point` 对象 `p1` 和 `p2`,并通过调用 `distance` 函数计算它们之间的距离,并将结果输出到控制台。
相关推荐
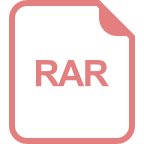
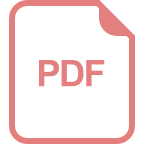
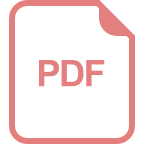
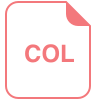
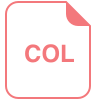










