PyQt5创建员工登录系统
时间: 2023-12-24 07:49:41 浏览: 74
以下是一个简单的员工登录系统的示例,使用PyQt5创建:
```python
from PyQt5.QtWidgets import QApplication, QWidget, QLabel, QLineEdit, QPushButton, QMessageBox
from PyQt5.QtGui import QIcon
import sys
class EmployeeLogin(QWidget):
def __init__(self):
super().__init__()
# 设置窗口标题、位置和大小
self.setWindowTitle('Employee Login')
self.setGeometry(450, 250, 350, 200)
self.setWindowIcon(QIcon('icon.png'))
# 创建标签、文本框和按钮
self.name_label = QLabel(self)
self.name_label.setText('Name:')
self.name_label.move(50, 30)
self.name_box = QLineEdit(self)
self.name_box.move(120, 30)
self.name_box.resize(200, 20)
self.password_label = QLabel(self)
self.password_label.setText('Password:')
self.password_label.move(50, 70)
self.password_box = QLineEdit(self)
self.password_box.setEchoMode(QLineEdit.Password)
self.password_box.move(120, 70)
self.password_box.resize(200, 20)
self.login_button = QPushButton('Login', self)
self.login_button.move(120, 110)
self.login_button.clicked.connect(self.login)
# 显示窗口
self.show()
def login(self):
# 获取用户名和密码
name = self.name_box.text()
password = self.password_box.text()
# 检查用户名和密码是否正确
if name == 'admin' and password == '1234':
QMessageBox.information(self, 'Success', 'Login Successful!')
else:
QMessageBox.warning(self, 'Error', 'Invalid Username or Password!')
if __name__ == '__main__':
app = QApplication(sys.argv)
ex = EmployeeLogin()
sys.exit(app.exec_())
```
这个示例创建了一个具有用户名和密码文本框以及一个登录按钮的窗口。当用户点击登录按钮时,程序将检查用户名和密码是否正确,并在成功或失败时显示相应的消息框。你可以根据需要修改此示例来满足自己的需求。
阅读全文
相关推荐
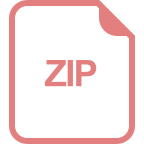

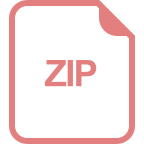
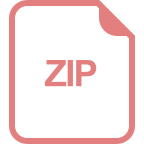
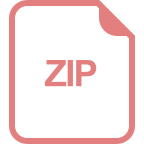
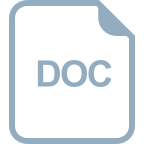
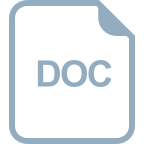
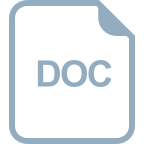
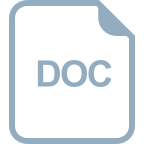
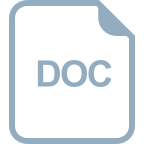
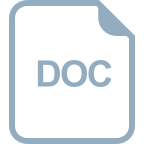
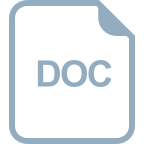
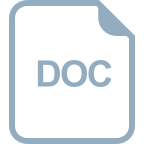
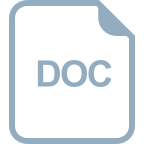
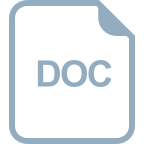
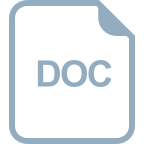
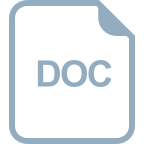