用eclipse写代码,将一个excel场位位置图表,生成一个平面网页,excel图表的内容中用行数和列数为坐标轴
时间: 2023-07-16 17:13:25 浏览: 167
要将一个Excel场位位置图表生成平面网页,可以通过以下步骤:
1. 使用Java代码读取Excel文件中的内容,可以使用POI库来实现。
2. 根据Excel表格中的行数和列数,计算出每个单元格在网页上的坐标值。
3. 将Excel表格中的内容转换为HTML格式的表格,并在对应的单元格中填入内容。
4. 使用HTML和CSS编写网页布局和样式。
下面是一个简单的实现示例:
```java
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.Workbook;
import org.apache.poi.ss.usermodel.WorkbookFactory;
public class ExcelToHtml {
public static void main(String[] args) throws IOException {
// 读取Excel文件
Workbook workbook = WorkbookFactory.create(new File("input.xlsx"));
Sheet sheet = workbook.getSheetAt(0);
// 计算单元格宽度和高度
int rowCount = sheet.getLastRowNum() + 1;
int colCount = sheet.getRow(0).getLastCellNum();
int cellWidth = 100 / colCount;
int cellHeight = 100 / rowCount;
// 生成HTML代码
StringBuilder html = new StringBuilder();
html.append("<table>");
for (int i = 0; i < rowCount; i++) {
html.append("<tr>");
for (int j = 0; j < colCount; j++) {
Cell cell = sheet.getRow(i).getCell(j);
String value = cell.getStringCellValue();
html.append("<td style=\"width: " + cellWidth + "%; height: " + cellHeight + "%;\">");
html.append(value);
html.append("</td>");
}
html.append("</tr>");
}
html.append("</table>");
// 保存为HTML文件
FileOutputStream fos = new FileOutputStream("output.html");
fos.write(html.toString().getBytes());
fos.close();
System.out.println("Convert successfully!");
}
}
```
该代码会将Excel文件中第一个Sheet中的内容转换为HTML格式,并保存为output.html文件。在HTML文件中,每个单元格的宽度和高度都根据表格的行数和列数计算出来,并且在对应的单元格中填入Excel表格中的内容。可以根据需要修改HTML和CSS代码来改变网页样式和布局。
阅读全文
相关推荐
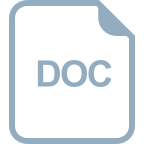
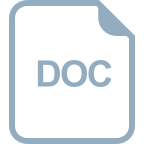
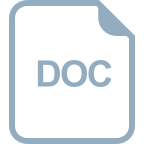
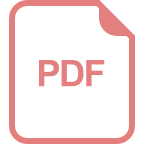
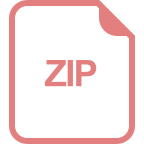
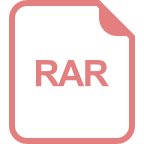
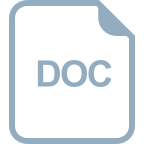
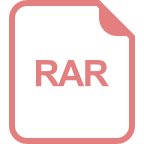
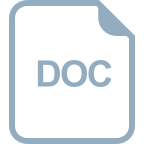
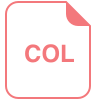
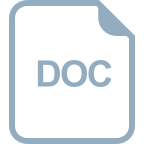
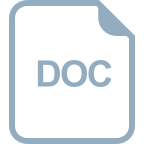
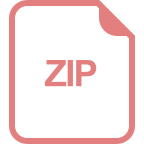
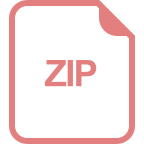
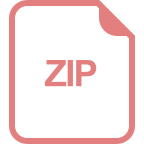
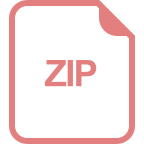
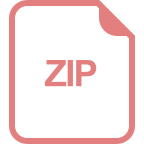