c# 使用opencv 展示mat对象 图片
时间: 2023-11-23 09:09:40 浏览: 134
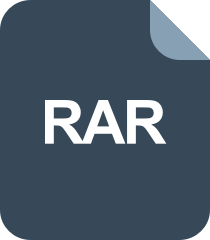
基于Opencv的图片显示

在 C# 中使用 OpenCV 展示 Mat 对象的图片需要借助于 OpenCV 的 C++ 接口,具体步骤如下:
1. 安装 OpenCV C++ 库,并将相关头文件和动态链接库添加到 C# 项目中。
2. 在 C# 代码中使用 `DllImport` 导入 OpenCV C++ 库中的 `imshow` 函数。
```csharp
[DllImport("opencv_world410.dll", CallingConvention = CallingConvention.Cdecl)]
private static extern void imshow(string winname, IntPtr mat);
```
3. 将 Mat 对象转换为 IntPtr 类型,并调用 `imshow` 函数显示图片。
```csharp
Mat image = new Mat("path/to/image.jpg", ImreadModes.Color);
IntPtr matPtr = image.CvPtr;
imshow("Window Name", matPtr);
```
完整的代码示例:
```csharp
using System;
using System.Runtime.InteropServices;
using OpenCvSharp;
namespace OpenCvSharpDemo
{
class Program
{
[DllImport("opencv_world410.dll", CallingConvention = CallingConvention.Cdecl)]
private static extern void imshow(string winname, IntPtr mat);
static void Main(string[] args)
{
Mat image = new Mat("path/to/image.jpg", ImreadModes.Color);
IntPtr matPtr = image.CvPtr;
imshow("Window Name", matPtr);
Cv2.WaitKey();
}
}
}
```
需要注意的是,由于 C# 和 C++ 使用不同的内存管理方式,因此需要手动释放 Mat 对象所占用的内存,避免内存泄漏。可以在程序结束时调用 `GC.Collect()` 和 `GC.WaitForPendingFinalizers()` 来释放内存。
阅读全文
相关推荐
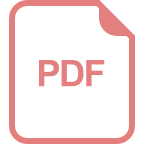
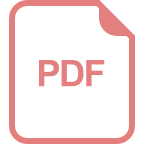
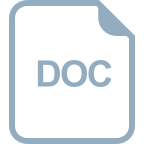
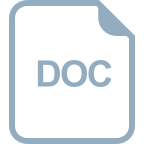











