opencv如何绘制旋转矩形
时间: 2023-11-03 07:04:43 浏览: 183
在OpenCV中,可以使用函数cv2.boxPoints()来获取一个旋转矩形的四个顶点坐标,然后使用函数cv2.drawContours()来绘制旋转矩形。
以下是一个示例代码:
```python
import cv2
import numpy as np
# 创建一个黑色的图像
img = np.zeros((512,512,3), np.uint8)
# 定义旋转矩形的参数
center = (256, 256)
size = (200, 100)
angle = 45
# 获取旋转矩形的四个顶点坐标
rect = cv2.boxPoints((center, size, angle))
rect = np.int0(rect)
# 绘制旋转矩形
cv2.drawContours(img, [rect], 0, (0, 255, 0), 2)
# 显示图像
cv2.imshow("Rotated Rectangle", img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
运行代码,将会在窗口中显示一个绘制了旋转矩形的黑色图像。
相关问题
python opencv获取旋转矩形
OpenCV提供了一个函数cv2.minAreaRect()来获取一个轮廓的最小外接矩形。这个函数返回的是一个Box2D结构体,其中包含了矩形的中心坐标、宽度、高度和旋转角度。下面是一个获取旋转矩形的代码示例:
```python
import cv2
# 读取图像并转为灰度图像
img = cv2.imread("test.jpg")
gray = cv2.cvtColor(img, cv2.COLOR_BGR2GRAY)
# 二值化图像
ret, binary = cv2.threshold(gray, 127, 255, cv2.THRESH_BINARY)
# 获取轮廓
contours, hierarchy = cv2.findContours(binary, cv2.RETR_TREE, cv2.CHAIN_APPROX_SIMPLE)
# 获取最小外接矩形
rect = cv2.minAreaRect(contours[0])
# 绘制旋转矩形
box = cv2.boxPoints(rect)
box = np.int0(box)
cv2.drawContours(img,[box],0,(0,0,255),2)
cv2.imshow("image", img)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
需要注意的是,cv2.minAreaRect()函数的参数是一个轮廓,所以在使用这个函数之前,需要先使用cv2.findContours()函数获取轮廓。另外,cv2.boxPoints()函数可以将旋转矩形转换为一个四个顶点的数组,方便绘制旋转矩形。
c++ opencv获取旋转矩形区域
在OpenCV中,如果你想要获取图像中的旋转矩形区域,你可以使用`getRotatedRect()`函数和`drawContours()`函数。首先,你需要找到轮廓(contours),这通常通过边缘检测(如Canny、HoughCircles或HoughLinesP等)或特征检测(如SIFT、SURF等)得到。
以下是一个简化的步骤:
1. **读取和预处理图像**:
```cpp
cv::Mat image = cv::imread("image.jpg", cv::IMREAD_GRAYSCALE);
```
2. **应用边缘检测或其他特征提取算法**:
```cpp
cv::Canny(image, edges, threshold1, threshold2);
std::vector<std::vector<cv::Point>> contours;
cv::findContours(edges, contours, cv::RETR_EXTERNAL, cv::CHAIN_APPROX_SIMPLE);
```
3. **寻找轮廓并获取旋转矩形**:
对于每个轮廓,可以这样计算旋转矩形:
```cpp
for (const auto& contour : contours) {
cv::Rect boundingRect = cv::boundingRect(contour);
cv::RotatedRect rotatedRect = cv::minAreaRect(contour); // 获取最小的旋转矩形
double angle = rotatedRect.angle; // 取得旋转角度
}
```
4. **绘制旋转矩形**:
```cpp
cv::Mat rotatedImage = cv::Mat::zeros(image.size(), CV_8UC3);
for (const auto& contour : contours) {
cv::drawContours(rotatedImage, {contour}, -1, Scalar(0, 255, 0), 2);
int left = rotatedRect.center.x - rotatedRect.size.width / 2;
int top = rotatedRect.center.y - rotatedRect.size.height / 2;
cv::rectangle(rotatedImage, Point(left, top), Point(left + rotatedRect.size.width, top + rotatedRect.size.height), Scalar(0, 0, 255), 2);
}
```
5. **显示结果**:
```cpp
cv::imshow("Original", image);
cv::imshow("Rotated Rectangles", rotatedImage);
```
阅读全文
相关推荐
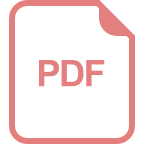



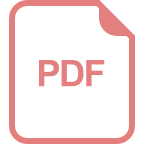


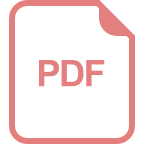
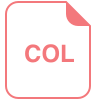
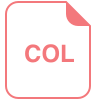



