Map<Character, Integer> count = new HashMap<>();
时间: 2023-12-11 16:02:26 浏览: 67
这段代码创建了一个 HashMap 对象,用于统计字符出现的次数。其中,键(key)表示字符,值(value)表示该字符出现的次数。具体来说,它可以用于遍历一个字符串,统计其中每个字符出现的次数,例如:
```
String str = "hello world";
for (int i = 0; i < str.length(); i++) {
char c = str.charAt(i);
count.put(c, count.getOrDefault(c, 0) + 1);
}
```
在这个例子中,我们遍历了字符串 "hello world",对于其中的每个字符,我们使用 `getOrDefault()` 方法获取其出现次数,如果该字符还没有出现过,则默认为 0,然后再加上 1,最后将其存入 HashMap 中。遍历完之后,`count` 就记录了每个字符出现的次数。
相关问题
public class IdiomProcessor { public static void main(String[] args) { // 定义输入文件路径和输出文件路径 String inputFile ="D:\yy\java\成语接龙\成语大全\去重后.txt"; String outputFile = "D:\yy\java\成语接龙\成语大全\全量成语处理后.txt"; try (BufferedReader reader = new BufferedReader(new FileReader(inputFile)); BufferedWriter writer = new BufferedWriter(new FileWriter(outputFile))) { // 定义成语列表和成语末尾字与开头字相同的数量Map List<String> idiomList = new ArrayList<>(); Map<Character, Integer> countMap = new HashMap<>(); // 读取每一行成语信息 String line; while ((line = reader.readLine())!= null) { if (line.trim().isEmpty()) { continue; // 跳过空行 } if (line.contains("拼音:")) { // 处理包含指定内容的行 System.out.println("我动了"); // 将成语与其他信息分割 String[] parts = line.split("拼音:"); // 将成语加入成语列表 String idiom = parts[0]; idiomList.add(idiom); // 计算成语末尾字与开头字相同的数量 char lastChar = idiom.charAt(idiom.length() - 1); countMap.put(lastChar, countMap.getOrDefault(lastChar, 0) + 1); // 将原来的信息每一条加上count后输出到另一个文件 String newLine = line + "可接:" + countMap.get(lastChar) + "\n"; writer.write(newLine); } } } catch (IOException e) { e.printStackTrace(); } } }由于代码在用Map统计lastChar时不同成语的末尾字会相同,所以用Map记录时,输出的count有错,如何改正
可以使用一个 `Map<String, Integer>` 来记录每个成语的末尾字与开头字相同的数量。
具体的做法是,在计算成语末尾字与开头字相同的数量时,将 `countMap` 改为 `idiomCountMap`,并且将 key 从 `Character` 改为 `String`,其 value 记录的是成语末尾字与开头字相同的数量。
修改后的代码如下:
```
public class IdiomProcessor {
public static void main(String[] args) {
// 定义输入文件路径和输出文件路径
String inputFile = "D:\\yy\\java\\成语接龙\\成语大全\\去重后.txt";
String outputFile = "D:\\yy\\java\\成语接龙\\成语大全\\全量成语处理后.txt";
try (BufferedReader reader = new BufferedReader(new FileReader(inputFile));
BufferedWriter writer = new BufferedWriter(new FileWriter(outputFile))) {
// 定义成语列表和成语末尾字与开头字相同的数量Map
List<String> idiomList = new ArrayList<>();
Map<String, Integer> idiomCountMap = new HashMap<>();
// 读取每一行成语信息
String line;
while ((line = reader.readLine()) != null) {
if (line.trim().isEmpty()) {
continue; // 跳过空行
}
if (line.contains("拼音:")) { // 处理包含指定内容的行
System.out.println("我动了");
// 将成语与其他信息分割
String[] parts = line.split("拼音:");
// 将成语加入成语列表
String idiom = parts[0];
idiomList.add(idiom);
// 计算成语末尾字与开头字相同的数量
char lastChar = idiom.charAt(idiom.length() - 1);
String key = lastChar + parts[1].substring(0, 1);
idiomCountMap.put(key, idiomCountMap.getOrDefault(key, 0) + 1);
// 将原来的信息每一条加上count后输出到另一个文件
String newLine = line + "可接:" + idiomCountMap.get(key) + "\n";
writer.write(newLine);
}
}
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
这样,就可以正确统计每个不同成语的末尾字与开头字相同的数量了。
public class IdiomProcessor { public static void main(String[] args) { try { FileReader fr = new FileReader("D:\yy\java\成语接龙\成语大全\去重后.txt"); BufferedReader br = new BufferedReader(fr); String line; HashMap<Character, ArrayList<String>> idiomMap = new HashMap<>(); while ((line = br.readLine()) != null) { String[] parts = line.trim().split("拼音:"); String idiom = parts[0]; //String info = parts[1]; if(!idiom.isEmpty()) { char tail = idiom.charAt(idiom.length() - 1); if (!idiomMap.containsKey(tail)) { idiomMap.put(tail, new ArrayList<String>()); } idiomMap.get(tail).add(idiom);} } br.close(); fr.close(); ArrayList<String> result = new ArrayList<>(); FileReader fr2 = new FileReader("D:\yy\java\成语接龙\成语大全\去重后.txt"); BufferedReader br2 = new BufferedReader(fr2); while ((line = br2.readLine()) != null) { String[] parts = line.trim().split("拼音:"); String idiom = parts[0]; //String info = parts[1]; if(!idiom.isEmpty()) { char tail = idiom.charAt(idiom.length() - 1); int count = idiomMap.get(tail).size() - 1; result.add(line.trim() + "可接:" + count); } } br2.close(); fr2.close(); FileWriter fw = new FileWriter("D:\yy\java\成语接龙\成语大全\全量成语处理后.txt"); BufferedWriter bw = new BufferedWriter(fw); for (String line2 : result) { bw.write(line2); bw.newLine(); } bw.close(); fw.close(); } catch (IOException e) { e.printStackTrace(); } } }请修改这个代码,改变count都相等的情况
可以尝试修改第二个while循环中的计算count的方式,具体方法如下:
1. 在第一个while循环中,使用另外一个HashMap来记录每个成语的尾字母出现的次数,键为成语的尾字母,值为对应的出现次数。
2. 在第二个while循环中,对于每个成语,先根据尾字母查找对应的成语列表,然后遍历该列表,统计其中可以接上该成语的个数。具体方法是,对于列表中的每个成语,将其尾字母作为键,查找对应的出现次数,将该出现次数作为可接个数。然后将所有可接个数相加,作为该成语的count值。
修改后的代码如下:
```java
public class IdiomProcessor {
public static void main(String[] args) {
try {
FileReader fr = new FileReader("D:\yy\java\成语接龙\成语大全\去重后.txt");
BufferedReader br = new BufferedReader(fr);
String line;
HashMap<Character, ArrayList<String>> idiomMap = new HashMap<>();
HashMap<Character, Integer> countMap = new HashMap<>(); // 新增计数Map
while ((line = br.readLine()) != null) {
String[] parts = line.trim().split("拼音:");
String idiom = parts[0];
if(!idiom.isEmpty()) {
char tail = idiom.charAt(idiom.length() - 1);
if (!idiomMap.containsKey(tail)) {
idiomMap.put(tail, new ArrayList<String>());
}
idiomMap.get(tail).add(idiom);
if(!countMap.containsKey(tail)) {
countMap.put(tail, 0);
}
countMap.put(tail, countMap.get(tail) + 1); // 尾字母计数加1
}
}
br.close();
fr.close();
ArrayList<String> result = new ArrayList<>();
FileReader fr2 = new FileReader("D:\yy\java\成语接龙\成语大全\去重后.txt");
BufferedReader br2 = new BufferedReader(fr2);
while ((line = br2.readLine()) != null) {
String[] parts = line.trim().split("拼音:");
String idiom = parts[0];
if(!idiom.isEmpty()) {
char tail = idiom.charAt(idiom.length() - 1);
ArrayList<String> idiomList = idiomMap.get(tail);
int count = 0;
for(String s : idiomList) {
if(s.equals(idiom)) continue; // 自己不能接自己
char first = s.charAt(0);
if(first == tail) {
count += countMap.get(first);
}
}
result.add(line.trim() + " 可接:" + count);
}
}
br2.close();
fr2.close();
FileWriter fw = new FileWriter("D:\yy\java\成语接龙\成语大全\全量成语处理后.txt");
BufferedWriter bw = new BufferedWriter(fw);
for (String line2 : result) {
bw.write(line2);
bw.newLine();
}
bw.close();
fw.close();
} catch (IOException e) {
e.printStackTrace();
}
}
}
```
相关推荐
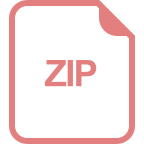
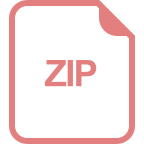
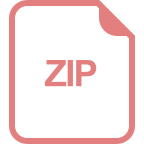












