c#中按照正则表达式替换
时间: 2023-07-11 14:28:51 浏览: 106
C#中可以使用`Regex.Replace()`方法按照正则表达式替换字符串,示例如下:
```csharp
string inputStr = "Hello, my name is John, and I am 25 years old.";
string pattern = @"(\d+)"; // 匹配数字
string replacement = "XX"; // 替换为"XX"
string outputStr = Regex.Replace(inputStr, pattern, replacement);
Console.WriteLine(outputStr); // 输出: "Hello, my name is John, and I am XX years old."
```
在上面的示例中,我们使用了正则表达式`(\d+)`,它表示匹配一个或多个数字。然后使用`Regex.Replace()`方法将匹配到的数字替换为"XX"。最终输出的字符串为"Hello, my name is John, and I am XX years old."。
需要注意的是,`Regex.Replace()`方法中第一个参数为待替换的字符串,第二个参数为正则表达式,第三个参数为替换后的字符串。如果想要在替换时引用正则表达式中的分组,可以使用`$1`、`$2`等符号来表示第1个、第2个分组等。
相关问题
什么是正则表达式?如何在C#中使用正则表达式?
正则表达式是一种用于匹配和操作字符串的强大工具。它是一种模式匹配的语法,可以用来检查字符串是否符合特定的模式,或者从字符串中提取出符合某种模式的部分。
在C#中,可以使用System.Text.RegularExpressions命名空间下的Regex类来使用正则表达式。以下是在C#中使用正则表达式的基本步骤:
1. 引入命名空间:
```csharp
using System.Text.RegularExpressions;
```
2. 创建正则表达式对象:
```csharp
Regex regex = new Regex(pattern);
```
其中,pattern是你要匹配的模式,可以使用正则表达式语法来定义。
3. 进行匹配操作:
```csharp
Match match = regex.Match(input);
```
其中,input是你要匹配的字符串。
4. 获取匹配结果:
```csharp
if (match.Success)
{
string result = match.Value;
// 对匹配结果进行处理
}
```
如果匹配成功,可以通过match.Value获取匹配到的字符串。
除了上述基本步骤外,还可以使用正则表达式的其他功能,如替换、分割等操作。可以通过Regex类提供的方法来实现。
C# 正则表达式替换
C#正则表达式替换是一种用于在字符串中查找并替换特定模式的功能。在C#中,可以使用System.Text.RegularExpressions命名空间中的Regex类来进行正则表达式的操作。
要进行替换操作,可以使用Regex类的静态方法Replace来实现。Replace方法接受三个参数:要进行替换的原始字符串、要匹配的正则表达式模式以及替换后的字符串。
下面是一个示例代码,演示了如何使用C#正则表达式替换:
```csharp
using System;
using System.Text.RegularExpressions;
class Program
{
static void Main()
{
string input = "Hello, world!";
string pattern = "world";
string replacement = "C#";
string result = Regex.Replace(input, pattern, replacement);
Console.WriteLine(result);
}
}
```
在上面的示例中,我们将字符串"Hello, world!"中的"world"替换为"C#",并将结果打印出来。输出结果为"Hello, C#!"。
阅读全文
相关推荐
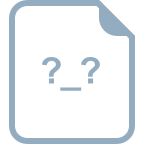
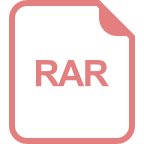
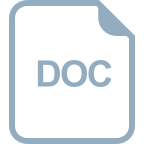
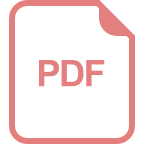
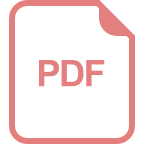
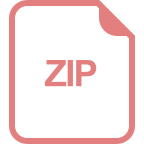
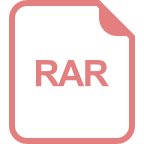
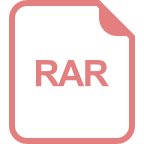
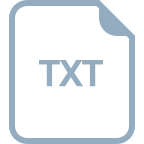
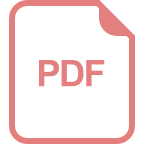
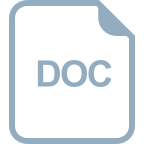