1.模拟实现一个简单的文本编辑器。 2.设计一个简单的文字输入的界面,并添加文字颜色和字号大小的编辑按钮。根据选择的不同按钮,显示出相应的效果。 3.除此之外,再添加一个滑块组件,根据滑块的位置变化,
时间: 2024-09-10 10:08:02 浏览: 72
要模拟实现一个简单的文本编辑器,我们需要考虑以下几个关键功能:
1. 文本的输入和显示区域,这是文本编辑器的核心,用户在这里输入文字。
2. 文字颜色和字号大小的编辑功能,需要提供按钮让用户选择不同的颜色和字号。
3. 滑块组件,用于调整某些属性,比如字体大小。
下面是一个简单的实现思路:
1. 文本输入和显示区域可以使用一个`EditText`(在Android中)或者一个`textarea`(在Web中)作为基础。
2. 文字颜色和字号大小的编辑功能,可以通过定义按钮与样式映射的关系,当用户点击不同的颜色或字号按钮时,触发相应的事件处理函数来改变文本区域的样式。
3. 对于滑块组件,可以用来动态调整字号大小。当滑块值改变时,根据滑块的值来更新文本区域的字号。
例如,在Android应用开发中,可以这样实现:
```java
public class SimpleTextEditorActivity extends AppCompatActivity {
private EditText editText;
private int currentFontSize = 16; // 默认字号大小
@Override
protected void onCreate(Bundle savedInstanceState) {
super.onCreate(savedInstanceState);
setContentView(R.layout.activity_simple_text_editor);
editText = findViewById(R.id.editText);
Button colorButton = findViewById(R.id.colorButton);
Button sizeButton = findViewById(R.id.sizeButton);
SeekBar sizeSeekBar = findViewById(R.id.sizeSeekBar);
colorButton.setOnClickListener(v -> {
// 弹出颜色选择对话框并设置选择的颜色
});
sizeButton.setOnClickListener(v -> {
// 切换字号大小按钮事件处理
currentFontSize += 2;
editText.setTextSize(TypedValue.COMPLEX_UNIT_SP, currentFontSize);
});
sizeSeekBar.setOnSeekBarChangeListener(new SeekBar.OnSeekBarChangeListener() {
@Override
public void onProgressChanged(SeekBar seekBar, int progress, boolean fromUser) {
// 根据滑块进度调整字号大小
currentFontSize = progress;
editText.setTextSize(TypedValue.COMPLEX_UNIT_SP, currentFontSize);
}
@Override
public void onStartTrackingTouch(SeekBar seekBar) {
// 滑块开始触摸的事件
}
@Override
public void onStopTrackingTouch(SeekBar seekBar) {
// 滑块停止触摸的事件
}
});
}
}
```
相应的XML布局文件可能包含以下控件:
```xml
<LinearLayout
android:layout_width="match_parent"
android:layout_height="match_parent"
android:orientation="vertical">
<EditText
android:id="@+id/editText"
android:layout_width="match_parent"
android:layout_height="0dp"
android:layout_weight="1"/>
<Button
android:id="@+id/colorButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Change Color"/>
<Button
android:id="@+id/sizeButton"
android:layout_width="wrap_content"
android:layout_height="wrap_content"
android:text="Change Size"/>
<SeekBar
android:id="@+id/sizeSeekBar"
android:layout_width="match_parent"
android:layout_height="wrap_content"/>
</LinearLayout>
```
阅读全文
相关推荐

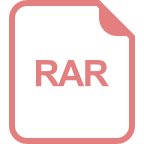















