基于DES的文件加/解密系统(CFB模式)C语言
时间: 2023-12-24 12:05:00 浏览: 133
以下是基于DES的文件加/解密系统(CFB模式)的C语言实现示例:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <openssl/des.h>
#define BUF_SIZE 1024
#define KEY_SIZE 8
#define IV_SIZE 8
void des_cfb_encryption(FILE *in, FILE *out, const unsigned char *key, const unsigned char *iv) {
DES_key_schedule schedule;
DES_set_key_checked(key, &schedule);
unsigned char ivec[IV_SIZE];
memcpy(ivec, iv, IV_SIZE);
unsigned char buffer[BUF_SIZE];
int num_read = 0;
while ((num_read = fread(buffer, sizeof(unsigned char), BUF_SIZE, in)) > 0) {
DES_cfb64_encrypt(buffer, buffer, num_read, &schedule, ivec, NULL, DES_ENCRYPT);
fwrite(buffer, sizeof(unsigned char), num_read, out);
}
}
void des_cfb_decryption(FILE *in, FILE *out, const unsigned char *key, const unsigned char *iv) {
DES_key_schedule schedule;
DES_set_key_checked(key, &schedule);
unsigned char ivec[IV_SIZE];
memcpy(ivec, iv, IV_SIZE);
unsigned char buffer[BUF_SIZE];
int num_read = 0;
while ((num_read = fread(buffer, sizeof(unsigned char), BUF_SIZE, in)) > 0) {
DES_cfb64_encrypt(buffer, buffer, num_read, &schedule, ivec, NULL, DES_DECRYPT);
fwrite(buffer, sizeof(unsigned char), num_read, out);
}
}
int main(int argc, char *argv[]) {
if (argc < 5) {
printf("Usage: %s <input_file> <output_file> <key> <iv>\n", argv[0]);
return 1;
}
FILE *in = fopen(argv[1], "rb");
if (!in) {
printf("Cannot open input file %s!\n", argv[1]);
return 1;
}
FILE *out = fopen(argv[2], "wb");
if (!out) {
printf("Cannot open output file %s!\n", argv[2]);
fclose(in);
return 1;
}
const char *key_str = argv[3];
if (strlen(key_str) != KEY_SIZE) {
printf("Invalid key size (%d bytes)!\n", (int)strlen(key_str));
fclose(in);
fclose(out);
return 1;
}
unsigned char key[KEY_SIZE];
memcpy(key, key_str, KEY_SIZE);
const char *iv_str = argv[4];
if (strlen(iv_str) != IV_SIZE) {
printf("Invalid IV size (%d bytes)!\n", (int)strlen(iv_str));
fclose(in);
fclose(out);
return 1;
}
unsigned char iv[IV_SIZE];
memcpy(iv, iv_str, IV_SIZE);
des_cfb_encryption(in, out, key, iv);
fclose(in);
fclose(out);
return 0;
}
```
在以上代码中,`des_cfb_encryption` 和 `des_cfb_decryption` 函数分别用于加密和解密文件,这两个函数的参数都包括输入文件、输出文件、密钥和初始化向量。其中,密钥和初始化向量都是8字节长度的。
在主函数中,首先打开输入和输出文件,然后解析命令行参数,将密钥和初始化向量转换成二进制格式,并调用 `des_cfb_encryption` 函数进行加密操作。最后关闭文件句柄并退出程序。
需要注意的是,这里使用了 OpenSSL 库中的 DES 实现。要编译以上代码,需要在命令行中添加 `-lcrypto` 选项,示例命令如下:
```bash
gcc -o des_cfb des_cfb.c -lcrypto
```
以上代码仅供参考,实际使用时需要根据自己的需求进行修改。
阅读全文
相关推荐
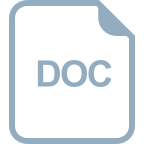
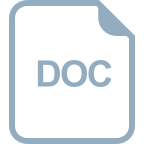
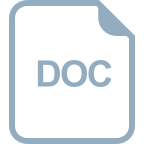

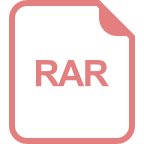
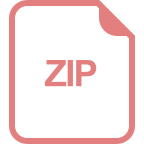
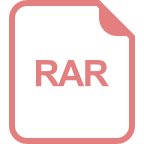
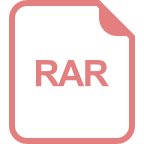
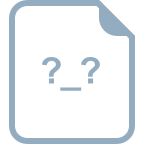
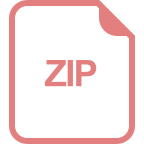
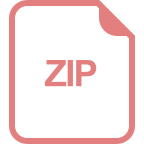
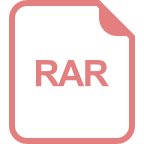
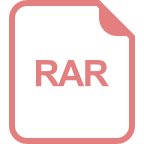
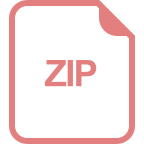
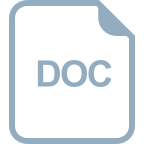
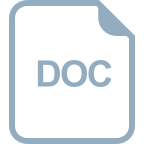
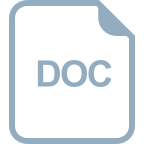
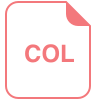
