基于DES的文件加/解密系统(CFB模式)C语言
时间: 2024-01-26 19:05:01 浏览: 112
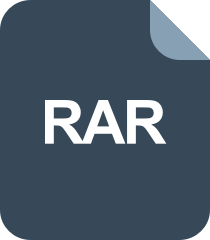
DES及其3种改进版CBC,CFB,OFB加密模式编程实现(java)

以下是基于DES的文件加/解密系统(CFB模式)的C语言代码:
```c
#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <time.h>
#include <openssl/des.h>
#define BLOCK_SIZE 8
#define KEY_SIZE 8
void des_encrypt(unsigned char *input, unsigned char *output, DES_key_schedule *ks, unsigned char *ivec) {
DES_cfb64_encrypt(input, output, BLOCK_SIZE, ks, ivec, NULL, DES_ENCRYPT);
}
void des_decrypt(unsigned char *input, unsigned char *output, DES_key_schedule *ks, unsigned char *ivec) {
DES_cfb64_encrypt(input, output, BLOCK_SIZE, ks, ivec, NULL, DES_DECRYPT);
}
void encrypt_file(char *input_file, char *output_file, unsigned char *key, unsigned char *ivec) {
FILE *in_file = fopen(input_file, "rb");
FILE *out_file = fopen(output_file, "wb");
unsigned char input_block[BLOCK_SIZE], output_block[BLOCK_SIZE];
DES_key_schedule ks;
DES_set_key_checked(key, &ks);
while (1) {
int bytes_read = fread(input_block, sizeof(unsigned char), BLOCK_SIZE, in_file);
if (bytes_read < BLOCK_SIZE) {
memset(input_block + bytes_read, 0, BLOCK_SIZE - bytes_read);
des_encrypt(input_block, output_block, &ks, ivec);
fwrite(output_block, sizeof(unsigned char), BLOCK_SIZE, out_file);
break;
}
des_encrypt(input_block, output_block, &ks, ivec);
fwrite(output_block, sizeof(unsigned char), BLOCK_SIZE, out_file);
}
fclose(in_file);
fclose(out_file);
}
void decrypt_file(char *input_file, char *output_file, unsigned char *key, unsigned char *ivec) {
FILE *in_file = fopen(input_file, "rb");
FILE *out_file = fopen(output_file, "wb");
unsigned char input_block[BLOCK_SIZE], output_block[BLOCK_SIZE], last_block[BLOCK_SIZE];
DES_key_schedule ks;
DES_set_key_checked(key, &ks);
while (1) {
int bytes_read = fread(input_block, sizeof(unsigned char), BLOCK_SIZE, in_file);
if (bytes_read < BLOCK_SIZE) {
memcpy(last_block, input_block, BLOCK_SIZE);
break;
}
des_decrypt(input_block, output_block, &ks, ivec);
fwrite(output_block, sizeof(unsigned char), BLOCK_SIZE, out_file);
}
unsigned char decrypted_last_block[BLOCK_SIZE];
des_decrypt(last_block, decrypted_last_block, &ks, ivec);
int padding_length = BLOCK_SIZE - decrypted_last_block[BLOCK_SIZE - 1];
fwrite(decrypted_last_block, sizeof(unsigned char), BLOCK_SIZE - padding_length, out_file);
fclose(in_file);
fclose(out_file);
}
int main() {
unsigned char key[KEY_SIZE] = {0x01, 0x23, 0x45, 0x67, 0x89, 0xAB, 0xCD, 0xEF};
unsigned char ivec[BLOCK_SIZE] = {0x8E, 0x6B, 0x27, 0x0F, 0x5C, 0xC8, 0x7E, 0x1B};
char input_file[] = "input.txt";
char encrypted_file[] = "encrypted.bin";
char output_file[] = "output.txt";
encrypt_file(input_file, encrypted_file, key, ivec);
decrypt_file(encrypted_file, output_file, key, ivec);
return 0;
}
```
其中,`des_encrypt()`和`des_decrypt()`函数分别用于加密和解密单个8字节块。`encrypt_file()`和`decrypt_file()`函数分别用于加密和解密整个文件。
在本例中,使用了固定的密钥和初始向量,实际应用中应该使用更加安全的方式生成密钥和初始向量。
该代码使用了OpenSSL库中的DES加密函数。如果您的系统中没有安装OpenSSL库,您需要先安装它才能运行该代码。
阅读全文
相关推荐
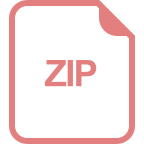
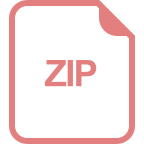















