基于AES的ESC与CFB加解密算法,用C++语言写
时间: 2024-10-13 09:11:22 浏览: 34
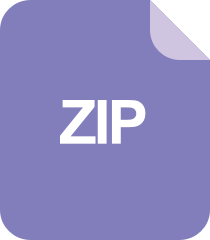
AES加解密算法(C++实现)
AES (Advanced Encryption Standard) 是一种高级加密标准,用于数据的安全传输。ESC (Electronic Codebook,电子密码本模式) 和 CFB (Cipher Feedback,反馈模式) 都是AES的两种常见加密模式。
ESC模式相当直接,它将明文块逐块地转换成密文块,每个明文块通过AES算法独立加密,不依赖前一个密文块,因此它的加密速度较快。然而,由于缺乏关联性,如果连续的数据块丢失,恢复会比较困难。
CFB模式则是一种流式加密模式,它利用前一密文块作为下一个密文块的输入,通过这种方式增加了一定的块间关联性,提供更好的安全性,适合处理连续的数据流。在解密时同样需要这个反馈链来还原原始信息。
以下是基于AES的ESC和CFB模式的简单C++加密示例(这里仅展示基本原理,实际应用中需引入库如 OpenSSL 或者 Crypto++,并注意内存管理和错误处理):
```cpp
#include <openssl/aes.h>
#include <cstring>
// AES key and IV setup
const unsigned char aes_key[] = "your_secret_key_32_bytes";
const unsigned char iv[] = "your_initialization_vector_16_bytes";
void encryptESC(const unsigned char* plaintext, size_t len, unsigned char* ciphertext) {
AES_KEY aesKey;
AES_set_encrypt_key(aes_key, 128, &aesKey); // 128 bits key
for (size_t i = 0; i < len; i += AES_BLOCK_SIZE) {
AES_encrypt(plaintext + i, ciphertext + i, &aesKey);
}
}
void encryptCFB(AES_KEY& aesKey, const unsigned char* plaintext, size_t len, unsigned char* ciphertext, const unsigned char* iv) {
AES.MODE_CFB;
AES_cbc_encrypt(plaintext, ciphertext, len, &aesKey, iv, AES_ENCRYPT);
}
// 示例用法
int main() {
unsigned char plaintext[AES_BLOCK_SIZE], ciphertext[AES_BLOCK_SIZE * 2];
// ...填充明文...
encryptESC(plaintext, sizeof(plaintext), ciphertext);
// ...存储并使用ciphertext...
// 对于CFB,需要先设置iv
encryptCFB(aesKey, plaintext, sizeof(plaintext), ciphertext, iv);
// ...后续操作同上...
return 0;
}
```
阅读全文
相关推荐
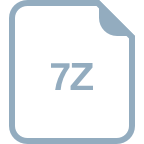
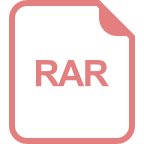

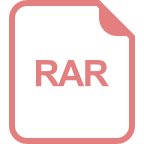
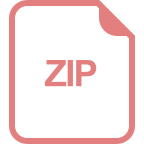
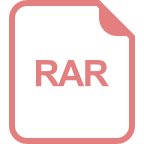
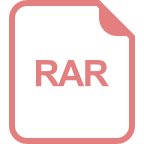
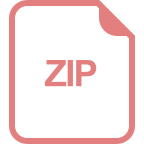
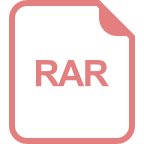
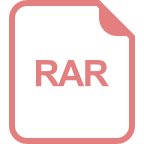
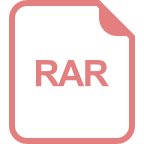
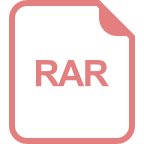
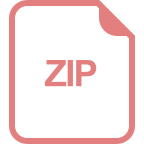
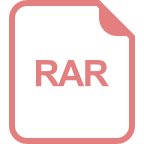
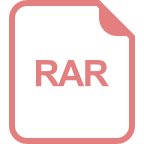
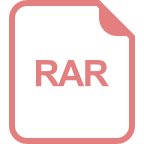
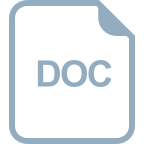