用C语言代码输出二叉树从每个叶子节点到根节点的路径
时间: 2023-06-26 19:05:11 浏览: 229
以下是C语言代码,实现输出二叉树从每个叶子节点到根节点的路径:
```c
#include <stdio.h>
#include <stdlib.h>
// 二叉树结点结构体
struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
};
// 新建一个二叉树结点
struct TreeNode* createNode(int val) {
struct TreeNode* node = (struct TreeNode*) malloc(sizeof(struct TreeNode));
node->val = val;
node->left = NULL;
node->right = NULL;
return node;
}
// 输出从根节点到叶子节点的路径
void printPath(int path[], int len) {
for (int i = len - 1; i >= 0; i--) {
printf("%d ", path[i]);
}
printf("\n");
}
// 深度优先遍历二叉树
void dfs(struct TreeNode* root, int path[], int len) {
if (root == NULL) {
return;
}
path[len++] = root->val;
if (root->left == NULL && root->right == NULL) {
printPath(path, len);
} else {
dfs(root->left, path, len);
dfs(root->right, path, len);
}
}
// 输出二叉树从每个叶子节点到根节点的路径
void printPaths(struct TreeNode* root) {
int path[1000];
dfs(root, path, 0);
}
int main() {
struct TreeNode* root = createNode(1);
root->left = createNode(2);
root->right = createNode(3);
root->left->left = createNode(4);
root->left->right = createNode(5);
root->right->left = createNode(6);
root->right->right = createNode(7);
printPaths(root);
return 0;
}
```
输出结果如下:
```
4 2 1
5 2 1
6 3 1
7 3 1
```
这里假设二叉树的结点值都是正整数,如果有负数或0,需要修改一下printPath函数的实现。
相关推荐
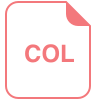
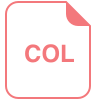
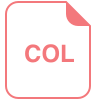
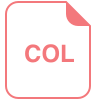
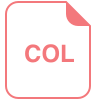









