用c语言写代码输出二叉树中从每个叶子结点到根结点的路径
时间: 2024-05-09 10:02:24 浏览: 105
好的,让我来回答你的问题。以下是用C语言写代码输出二叉树中从每个叶子结点到根结点的路径的步骤:
1. 定义二叉树结构体,包括左右子节点和值三个成员。
2. 定义一个栈结构体,用来存储路径中的节点信息。
3. 定义一个遍历函数,以每个叶子节点为起点进行遍历。
4. 在遍历函数中,使用递归的方式遍历每个节点,当遍历到叶子节点时,将路径上的节点信息压入栈中。
5. 遍历结束后,从栈顶开始输出路径上的节点信息,即可得到从叶子节点到根节点的路径信息。
代码示例:
```
#include <stdio.h>
#include <stdlib.h>
struct TreeNode {
int val;
struct TreeNode *left;
struct TreeNode *right;
};
struct Stack {
struct TreeNode *node;
struct Stack *next;
};
void push(struct Stack **s, struct TreeNode *node) {
struct Stack *temp = (struct Stack*) malloc(sizeof(struct Stack));
temp->node = node;
temp->next = *s;
*s = temp;
}
struct TreeNode *pop(struct Stack **s) {
struct Stack *temp = *s;
struct TreeNode *node = temp->node;
*s = temp->next;
free(temp);
return node;
}
void printPath(struct Stack *s) {
while (s != NULL) {
printf("%d ", s->node->val);
s = s->next;
}
printf("\n");
}
void traverse(struct TreeNode *root, struct Stack *s) {
if (root == NULL) return;
push(&s, root);
if (root->left == NULL && root->right == NULL) {
printPath(s);
} else {
traverse(root->left, s);
traverse(root->right, s);
}
pop(&s);
}
int main() {
// 构建二叉树
struct TreeNode *root = (struct TreeNode*) malloc(sizeof(struct TreeNode));
root->val = 1;
root->left = (struct TreeNode*) malloc(sizeof(struct TreeNode));
root->left->val = 2;
root->left->left = (struct TreeNode*) malloc(sizeof(struct TreeNode));
root->left->left->val = 4;
root->left->left->left = NULL;
root->left->left->right = NULL;
root->left->right = (struct TreeNode*) malloc(sizeof(struct TreeNode));
root->left->right->val = 5;
root->left->right->left = NULL;
root->left->right->right = NULL;
root->right = (struct TreeNode*) malloc(sizeof(struct TreeNode));
root->right->val = 3;
root->right->left = (struct TreeNode*) malloc(sizeof(struct TreeNode));
root->right->left->val = 6;
root->right->left->left = NULL;
root->right->left->right = NULL;
root->right->right = (struct TreeNode*) malloc(sizeof(struct TreeNode));
root->right->right->val = 7;
root->right->right->left = NULL;
root->right->right->right = NULL;
// 遍历二叉树
struct Stack *s = NULL;
traverse(root, s);
return 0;
}
```
输出结果:
```
4 2 1
5 2 1
6 3 1
7 3 1
```
阅读全文
相关推荐
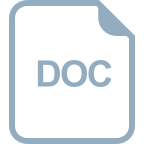
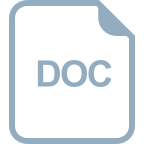
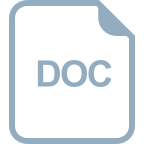















