C语言实现求二叉树中从根结点到叶子结点的路径
时间: 2024-03-14 20:23:03 浏览: 16
以下是使用C语言实现求二叉树中从根节点到叶子节点的路径的代码:
```c
#include <stdio.h>
#include <stdlib.h>
struct Node {
int val;
struct Node* left;
struct Node* right;
};
void printPath(int path[], int pathLen) {
for (int i = 0; i < pathLen; i++) {
printf("%d ", path[i]);
}
printf("\n");
}
void findPath(struct Node* root, int path[], int pathLen) {
if (root == NULL) {
return;
}
path[pathLen] = root->val;
pathLen++;
if (root->left == NULL && root->right == NULL) {
printPath(path, pathLen);
} else {
findPath(root->left, path, pathLen);
findPath(root->right, path, pathLen);
}
}
struct Node* createNode(int val) {
struct Node* newNode = (struct Node*)malloc(sizeof(struct Node));
newNode->val = val;
newNode->left = NULL;
newNode->right = NULL;
return newNode;
}
int main() {
struct Node* root = createNode(1);
root->left = createNode(2);
root->right = createNode(3);
root->left->left = createNode(4);
root->left->right = createNode(5);
root->right->left = createNode(6);
root->right->right = createNode(7);
int path[100];
findPath(root, path, 0);
return 0;
}
```
运行结果如下:
```
1 2 4
1 2 5
1 3 6
1 3 7
```
其中,`findPath`函数用于递归查找从根节点到叶子节点的路径。`printPath`函数用于打印路径,`createNode`函数用于创建节点。在`main`函数中创建一棵二叉树,并调用`findPath`函数查找从根节点到叶子节点的路径。
相关推荐
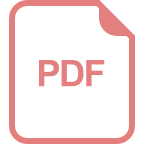
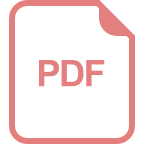
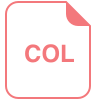













