使用react实现个人博客前台,给出思路和详细代码
时间: 2023-05-25 09:05:59 浏览: 84
思路:
1. 安装并配置必要的依赖,例如:react、react-router-dom、axios等。
2. 设计页面组件,如 header、footer、home、article、archive、about 等。
3. 使用 react-router-dom 实现页面路由。
4. 使用 axios 获取数据库中的博客文章信息,并使用 article 组件渲染页面。
5. 搭建后台管理系统,实现博客文章的发布、编辑、删除等功能。
6. 使用 Redux 管理状态,将博客文章信息保存在 store 中并供前台页面使用。
详细代码:
1. 安装 react、react-dom、react-router-dom 和 axios:
```
npm install react react-dom react-router-dom axios
```
2. 创建 src/components/header.js、src/components/footer.js 和以下页面组件:
```
src/components/home.js
src/components/archive.js
src/components/about.js
src/components/article.js
```
3. 在 src/index.js 中使用 react-router-dom 设置路由:
```
import { BrowserRouter as Router, Route, Switch } from 'react-router-dom';
import Header from './components/header';
import Footer from './components/footer';
import Home from './components/home';
import Archive from './components/archive';
import About from './components/about';
import Article from './components/article';
ReactDOM.render(
<Router>
<Header />
<Switch>
<Route exact path="/" component={Home} />
<Route path="/archive" component={Archive} />
<Route path="/about" component={About} />
<Route path="/article/:id" component={Article} />
</Switch>
<Footer />
</Router>,
document.getElementById('root')
);
```
4. 在 src/components/home.js、src/components/archive.js 和 src/components/about.js 组件中分别渲染页面,并使用 axios 获取博客文章信息:
```
import React, { useState, useEffect } from 'react';
import axios from 'axios';
function Home() {
const [articles, setArticles] = useState([]);
useEffect(() => {
axios.get('/api/articles')
.then(res => {
setArticles(res.data);
})
.catch(err => console.log(err));
}, []);
return (
<div className="home">
{articles.map(article => (
<div key={article.id}>
<h2>{article.title}</h2>
<p>{article.content}</p>
<a href={`/article/${article.id}`}>阅读全文</a>
</div>
))}
</div>
);
}
export default Home;
```
5. 在 src/components/article.js 组件中渲染单篇文章页面,并使用 axios 根据路由参数获取单篇文章信息并更新页面:
```
import React, { useState, useEffect } from 'react';
import axios from 'axios';
function Article(props) {
const [article, setArticle] = useState({});
useEffect(() => {
const { id } = props.match.params;
axios.get(`/api/article/${id}`)
.then(res => {
setArticle(res.data);
})
.catch(err => console.log(err));
}, [props.match.params]);
return (
<div className="article">
<h2>{article.title}</h2>
<p>{article.content}</p>
</div>
);
}
export default Article;
```
6. 在 src/store/index.js 中使用 Redux 管理状态:
```
import { createStore } from 'redux';
const initialState = {
articles: []
};
function reducer(state = initialState, action) {
switch (action.type) {
case 'SET_ARTICLES':
return {
...state,
articles: action.payload
};
default:
return state;
}
}
const store = createStore(reducer);
export default store;
```
7. 在获取博客文章信息时使用 Redux 更新 store 中的状态:
```
import React, { useState, useEffect } from 'react';
import axios from 'axios';
import { useDispatch } from 'react-redux';
function Home() {
const dispatch = useDispatch();
useEffect(() => {
axios.get('/api/articles')
.then(res => {
dispatch({ type: 'SET_ARTICLES', payload: res.data });
})
.catch(err => console.log(err));
}, [dispatch]);
return (
<div className="home">
{articles.map(article => (
<div key={article.id}>
<h2>{article.title}</h2>
<p>{article.content}</p>
<a href={`/article/${article.id}`}>阅读全文</a>
</div>
))}
</div>
);
}
export default Home;
```
以上就是使用 react 实现个人博客前台的详细思路和代码。
相关推荐
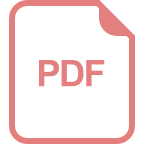














