C++具有串口监控功能,可以监控、拦截、保存所收发的数据的demo
时间: 2024-03-12 19:50:07 浏览: 77
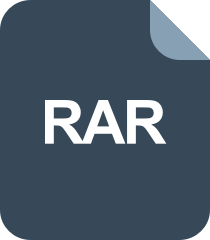
c++ 串口开发 发送/接受数据demo
在 C++ 中,您可以使用 WinAPI 提供的串口通讯函数和钩子函数来实现串口的监控和拦截。下面是一个简单的示例代码:
```c++
#include <Windows.h>
#include <iostream>
#include <fstream>
HHOOK hHook = NULL;
HANDLE hComm = NULL;
DCB dcb;
COMMTIMEOUTS timeouts;
std::ofstream outfile("log.txt");
LRESULT CALLBACK SerialProc(int nCode, WPARAM wParam, LPARAM lParam)
{
if (nCode == HC_ACTION && wParam == EV_RXCHAR)
{
DWORD dwBytesRead;
char buffer[1024];
ReadFile(hComm, buffer, sizeof(buffer), &dwBytesRead, NULL);
if (dwBytesRead > 0)
{
outfile.write(buffer, dwBytesRead);
outfile.flush();
}
}
return CallNextHookEx(hHook, nCode, wParam, lParam);
}
int main()
{
hComm = CreateFile("COM1", GENERIC_READ | GENERIC_WRITE, 0, NULL, OPEN_EXISTING, 0, NULL);
if (hComm == INVALID_HANDLE_VALUE)
{
std::cerr << "Failed to open COM1" << std::endl;
return 1;
}
if (!GetCommState(hComm, &dcb))
{
std::cerr << "Failed to get DCB" << std::endl;
CloseHandle(hComm);
return 1;
}
dcb.BaudRate = CBR_9600;
dcb.ByteSize = 8;
dcb.Parity = NOPARITY;
dcb.StopBits = ONESTOPBIT;
if (!SetCommState(hComm, &dcb))
{
std::cerr << "Failed to set DCB" << std::endl;
CloseHandle(hComm);
return 1;
}
timeouts.ReadIntervalTimeout = MAXDWORD;
timeouts.ReadTotalTimeoutMultiplier = 0;
timeouts.ReadTotalTimeoutConstant = 0;
timeouts.WriteTotalTimeoutMultiplier = 0;
timeouts.WriteTotalTimeoutConstant = 0;
if (!SetCommTimeouts(hComm, &timeouts))
{
std::cerr << "Failed to set timeouts" << std::endl;
CloseHandle(hComm);
return 1;
}
hHook = SetWindowsHookEx(WH_GETMESSAGE, SerialProc, NULL, GetCurrentThreadId());
MSG msg;
while (GetMessage(&msg, NULL, 0, 0) > 0)
{
TranslateMessage(&msg);
DispatchMessage(&msg);
}
UnhookWindowsHookEx(hHook);
outfile.close();
CloseHandle(hComm);
return 0;
}
```
这段代码可以打开串口 `COM1`,设置波特率、数据位、停止位和校验位,然后使用钩子函数监控串口接收数据的事件,并将收到的数据保存在 `log.txt` 文件中。您可以根据自己的需要修改代码,实现串口的监控和拦截,并将收发的数据保存在文件中。需要注意的是,钩子函数需要运行在一个独立的线程中,否则可能会阻塞主线程的消息循环。另外,您需要在程序中打开和关闭串口,并正确设置串口参数和超时时间,以保证串口通信的稳定性。
阅读全文
相关推荐

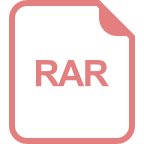
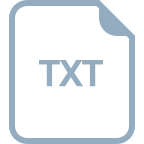
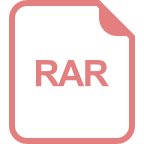
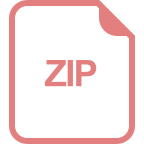
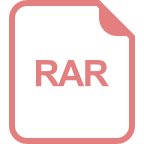
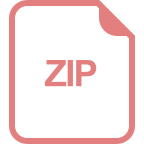
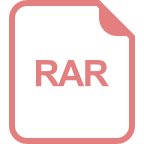
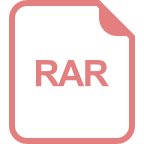
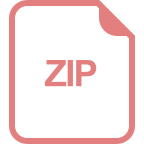
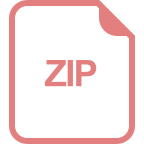