python实现ripley's k 函数
时间: 2023-11-07 19:04:47 浏览: 95
Ripley's K 函数是用来对点模式进行空间分布分析的一种方法,它可以用来判断点模式的随机性和聚集性。下面是一个 Python 实现 Ripley's K 函数的示例代码:
```python
import numpy as np
import matplotlib.pyplot as plt
def ripley_k(points, r_max, n_trials=50):
"""
Computes Ripley's K function for a set of 2D points.
Parameters:
points: numpy array, shape (n_points, 2)
The x, y coordinates of the points.
r_max: float
The maximum distance at which to compute K.
n_trials: int, optional
The number of random trials to use for estimating the expected K function.
Returns:
r: numpy array, shape (n_bins,)
The distances at which K was computed.
k: numpy array, shape (n_bins,)
The values of K at each distance.
k_expected: numpy array, shape (n_bins,)
The expected values of K at each distance (computed by averaging over n_trials random trials).
"""
n_points = len(points)
r_bins = np.linspace(0, r_max, 50)
dr = r_bins[1] - r_bins[0]
area = np.pi * r_max**2
k = np.zeros(len(r_bins))
k_expected = np.zeros(len(r_bins))
for i in range(n_points):
d = np.linalg.norm(points[i] - points, axis=1)
histogram, _ = np.histogram(d, bins=r_bins)
k += histogram / (n_points * area)
for i in range(n_trials):
random_points = np.random.rand(n_points, 2) * r_max
d = np.linalg.norm(random_points[:, None] - random_points, axis=2)
histogram, _ = np.histogram(d, bins=r_bins)
k_expected += histogram / (n_points * area)
k_expected /= n_trials
r = r_bins[:-1] + dr/2
return r, k, k_expected
```
这个函数接受一个包含 2D 点坐标的 numpy 数组,以及一个最大距离 r_max。它会计算在每个距离上点的聚集程度,并返回一个包含距离、K 值和期望 K 值的 numpy 数组。在计算期望 K 值时,它会进行 n_trials 次随机实验,并取平均值作为期望值。你可以使用 Matplotlib 库将 K 函数可视化,例如:
```python
points = np.random.rand(100, 2) * 10
r, k, k_expected = ripley_k(points, 5)
plt.plot(r, k)
plt.plot(r, k_expected, '--')
plt.xlabel('Distance')
plt.ylabel('K function')
plt.show()
```
这段代码会生成一个包含实际 K 函数和期望 K 函数的图表。
相关推荐
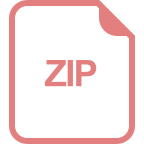
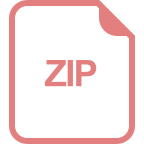












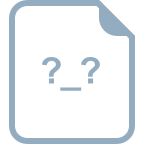
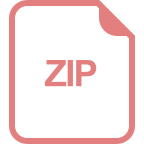
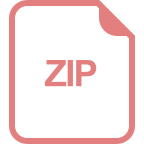