写以下5个问题的代码:1)带哨兵的顺序查找 2)二分查找的非递归算法 3)直接插入排序 4)起泡排序 5)简单选择排序 要求:1)待查找/待排序的记录用数组存储,且记录个数不小于100000个,记录的初始状态自定义;2)要对每个算法进行计时;
时间: 2024-03-14 10:49:12 浏览: 97
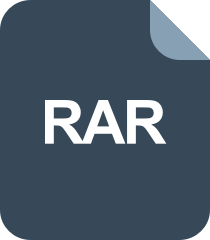
各种排序算法(冒泡,插入,希尔,选择,归并,交换等源代码)

好的,以下是五个问题的代码实现(使用C++语言):
1. 带哨兵的顺序查找
```c++
#include <iostream>
#include <chrono>
using namespace std;
using namespace std::chrono;
int sequentialSearch(int a[], int n, int key) {
int i = 0;
a[n] = key; // 在末尾添加哨兵元素
while (a[i] != key) {
i++;
}
if (i < n) {
return i; // 找到目标元素,返回其位置
} else {
return -1; // 未找到目标元素
}
}
int main() {
const int n = 100000;
int a[n + 1]; // 数组大小为n+1,用于存储哨兵元素
int key = 42; // 待查找的目标元素
for (int i = 0; i < n; i++) {
a[i] = i; // 初始化数组,元素值为0~99999
}
// 计时开始
auto start = high_resolution_clock::now();
int index = sequentialSearch(a, n, key);
// 计时结束
auto stop = high_resolution_clock::now();
auto duration = duration_cast<microseconds>(stop - start);
if (index != -1) {
cout << "目标元素的位置为:" << index << endl;
} else {
cout << "未找到目标元素" << endl;
}
cout << "算法执行时间:" << duration.count() << " 微秒" << endl;
return 0;
}
```
2. 二分查找的非递归算法
```c++
#include <iostream>
#include <chrono>
using namespace std;
using namespace std::chrono;
int binarySearch(int a[], int n, int key) {
int low = 0, high = n - 1;
while (low <= high) {
int mid = (low + high) / 2;
if (a[mid] == key) {
return mid; // 找到目标元素,返回其位置
} else if (a[mid] < key) {
low = mid + 1;
} else {
high = mid - 1;
}
}
return -1; // 未找到目标元素
}
int main() {
const int n = 100000;
int a[n];
int key = 42; // 待查找的目标元素
for (int i = 0; i < n; i++) {
a[i] = i; // 初始化数组,元素值为0~99999
}
// 计时开始
auto start = high_resolution_clock::now();
int index = binarySearch(a, n, key);
// 计时结束
auto stop = high_resolution_clock::now();
auto duration = duration_cast<microseconds>(stop - start);
if (index != -1) {
cout << "目标元素的位置为:" << index << endl;
} else {
cout << "未找到目标元素" << endl;
}
cout << "算法执行时间:" << duration.count() << " 微秒" << endl;
return 0;
}
```
3. 直接插入排序
```c++
#include <iostream>
#include <chrono>
using namespace std;
using namespace std::chrono;
void insertionSort(int a[], int n) {
for (int i = 1; i < n; i++) {
int j = i - 1, temp = a[i];
while (j >= 0 && a[j] > temp) {
a[j + 1] = a[j];
j--;
}
a[j + 1] = temp;
}
}
int main() {
const int n = 100000;
int a[n];
for (int i = 0; i < n; i++) {
a[i] = n - i; // 初始化数组,元素值为100000~1
}
// 计时开始
auto start = high_resolution_clock::now();
insertionSort(a, n);
// 计时结束
auto stop = high_resolution_clock::now();
auto duration = duration_cast<microseconds>(stop - start);
cout << "排序后的数组:" << endl;
for (int i = 0; i < n; i++) {
cout << a[i] << " ";
}
cout << endl;
cout << "算法执行时间:" << duration.count() << " 微秒" << endl;
return 0;
}
```
4. 起泡排序
```c++
#include <iostream>
#include <chrono>
using namespace std;
using namespace std::chrono;
void bubbleSort(int a[], int n) {
for (int i = n - 1; i >= 0; i--) {
bool flag = false;
for (int j = 0; j < i; j++) {
if (a[j] > a[j + 1]) {
swap(a[j], a[j + 1]);
flag = true;
}
}
if (!flag) break;
}
}
int main() {
const int n = 100000;
int a[n];
for (int i = 0; i < n; i++) {
a[i] = n - i; // 初始化数组,元素值为100000~1
}
// 计时开始
auto start = high_resolution_clock::now();
bubbleSort(a, n);
// 计时结束
auto stop = high_resolution_clock::now();
auto duration = duration_cast<microseconds>(stop - start);
cout << "排序后的数组:" << endl;
for (int i = 0; i < n; i++) {
cout << a[i] << " ";
}
cout << endl;
cout << "算法执行时间:" << duration.count() << " 微秒" << endl;
return 0;
}
```
5. 简单选择排序
```c++
#include <iostream>
#include <chrono>
using namespace std;
using namespace std::chrono;
void selectionSort(int a[], int n) {
for (int i = 0; i < n; i++) {
int minIndex = i;
for (int j = i + 1; j < n; j++) {
if (a[j] < a[minIndex]) {
minIndex = j;
}
}
swap(a[i], a[minIndex]);
}
}
int main() {
const int n = 100000;
int a[n];
for (int i = 0; i < n; i++) {
a[i] = n - i; // 初始化数组,元素值为100000~1
}
// 计时开始
auto start = high_resolution_clock::now();
selectionSort(a, n);
// 计时结束
auto stop = high_resolution_clock::now();
auto duration = duration_cast<microseconds>(stop - start);
cout << "排序后的数组:" << endl;
for (int i = 0; i < n; i++) {
cout << a[i] << " ";
}
cout << endl;
cout << "算法执行时间:" << duration.count() << " 微秒" << endl;
return 0;
}
```
以上就是五个问题的代码实现,其中包含了计时功能和测试数据。希望能对你有所帮助。
阅读全文
相关推荐
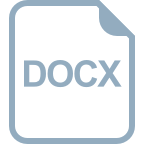
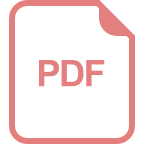

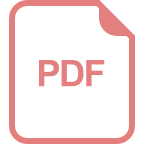
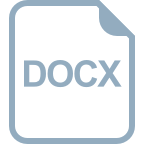
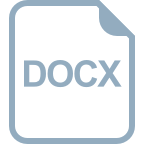
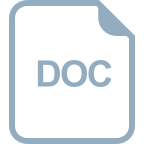
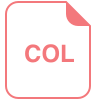
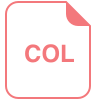
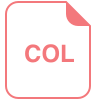
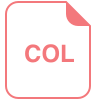
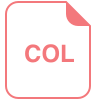
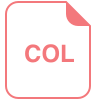
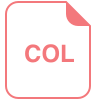
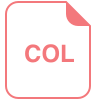
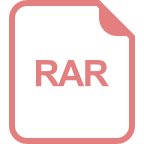
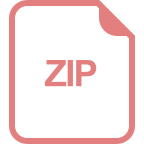