opencv 对彩色图片进行LSC c++代码
时间: 2023-07-24 17:33:29 浏览: 130
以下是使用OpenCV对彩色图像进行LSC的C++代码示例:
```c++
#include <iostream>
#include <opencv2/opencv.hpp>
using namespace cv;
using namespace std;
int main() {
// 加载彩色图像
Mat src = imread("input.jpg");
if (src.empty()) {
cerr << "Failed to load input image!" << endl;
return -1;
}
// 将彩色图像转换为灰度图像
Mat gray;
cvtColor(src, gray, COLOR_BGR2GRAY);
// 计算灰度图像的直方图
Mat hist;
int histSize = 256;
float range[] = {0, 256};
const float *ranges[] = {range};
calcHist(&gray, 1, 0, Mat(), hist, 1, &histSize, ranges);
// 计算直方图的累积分布函数
Mat cdf;
hist.copyTo(cdf);
for (int i = 1; i < histSize; ++i) {
cdf.at<float>(i) += cdf.at<float>(i - 1);
}
cdf /= gray.total();
// 计算LSC映射表
Mat lut(1, histSize, CV_8U);
float alpha = 255.0 / (gray.total() - cdf.at<float>(0));
for (int i = 0; i < histSize; ++i) {
lut.at<uchar>(i) = saturate_cast<uchar>(alpha * (cdf.at<float>(i) - cdf.at<float>(0)));
}
// 对彩色图像的三个通道分别进行LSC处理
vector<Mat> bgr;
split(src, bgr);
for (int i = 0; i < 3; ++i) {
LUT(bgr[i], lut, bgr[i]);
}
Mat dst;
merge(bgr, dst);
// 显示结果
imshow("Input", src);
imshow("Output", dst);
waitKey(0);
return 0;
}
```
需要注意的是,LSC是一种直方图均衡化方法,会增加图像的对比度和亮度,可能会导致图像失真。在实际应用中,需要根据具体情况选择合适的直方图均衡化方法。
阅读全文
相关推荐
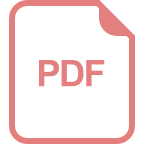
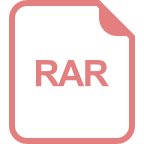
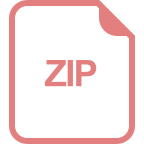
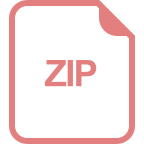
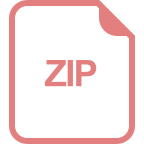
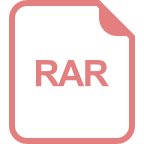
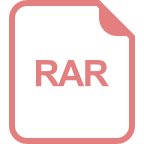
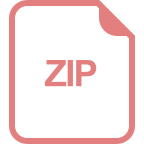
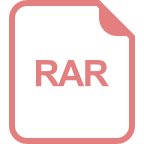
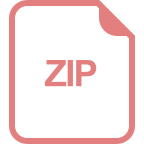
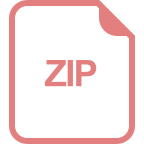
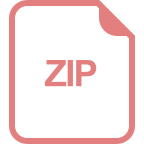