C++中如何实现类似的需求?
时间: 2024-10-13 07:15:09 浏览: 20
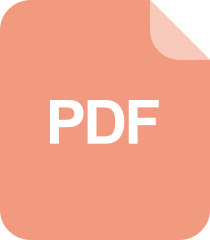
C++中接口的实现
在C++中,如果你想要实现一个表达式必须为整数或未指定范围的枚举类型,你可以使用标准库中的`std::variant`或者直接使用`enum class`配合`static_assert`来进行类型检查。这里以`enum class`为例说明:
```cpp
#include <iostream>
#include <type_traits>
// 创建一个枚举类,如果没有范围,则所有枚举项都是等价的
enum class MyUnspecifiedEnum { Value1, Value2 };
enum class MyIntegerEnum {
IntegerValue1,
IntegerValue2
};
// 类型别名用于简化检查
using IntegerOrEnum = std::variant<int, MyUnspecifiedEnum, MyIntegerEnum>;
void processExpression(IntegerOrEnum expr) {
if constexpr (std::is_same_v<decltype(expr), int>) {
std::cout << "处理整数:" << expr << std::endl;
} else if constexpr (std::is_same_v<decltype(expr), MyUnspecifiedEnum>) {
std::cout << "处理未指定范围的枚举:" << static_cast<MyUnspecifiedEnum>(expr) << std::endl;
} else if constexpr (std::is_same_v<decltype(expr), MyIntegerEnum>) {
std::cout << "处理整数枚举:" << static_cast<MyIntegerEnum>(expr) << std::endl;
} else {
std::cerr << "无效的表达式类型!" << std::endl;
}
}
int main() {
processExpression(10); // 整数示例
processExpression(MyUnspecifiedEnum::Value1); // 未指定范围的枚举示例
processExpression(MyIntegerEnum::IntegerValue1); // 整数枚举示例
// 不合法的类型会被编译器阻止
processExpression(std::string("Invalid")); // 这将导致编译错误
return 0;
}
```
在这个例子中,`processExpression`函数接受一个`IntegerOrEnum`类型的变量,通过`constexpr`和`std::is_same_v`判断表达式的类型。如果尝试传递非预期类型,编译器会在编译阶段给出错误。
阅读全文
相关推荐
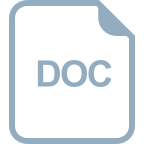
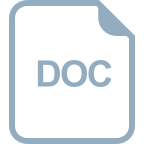
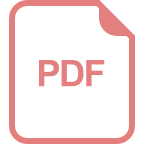
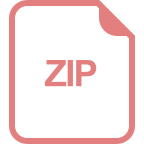
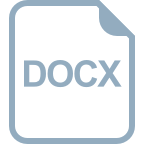
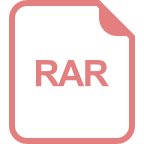
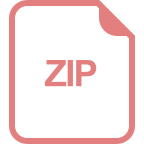
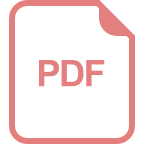
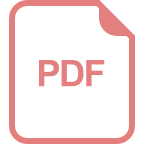
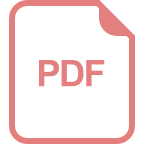
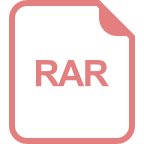
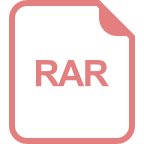
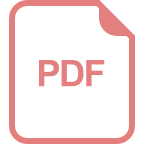
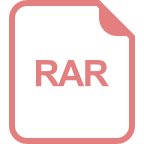
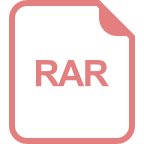
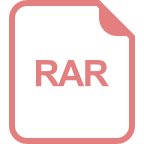
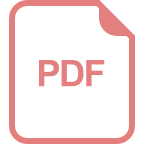
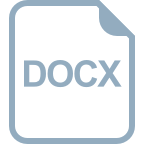