熵值法 python
时间: 2024-04-06 17:27:34 浏览: 108
熵值法(Entropy Weight Method)是一种常用的多指标综合评价方法,用于确定各指标的权重。在Python中,可以使用一些库来实现熵值法。
一种常用的库是`numpy`,它提供了一些计算熵值法的函数。下面是一个简单的示例代码,演示如何使用熵值法计算指标的权重:
```python
import numpy as np
# 假设有3个指标,每个指标有4个候选方案
matrix = np.array([
[1, 2, 3, 4],
[4, 3, 2, 1],
[2, 4, 1, 3]
])
# 标准化矩阵
normalized_matrix = matrix / matrix.sum(axis=0)
# 计算熵值
entropy = -np.sum(normalized_matrix * np.log(normalized_matrix), axis=0)
# 计算权重
weights = (1 - entropy) / np.sum(1 - entropy)
print("指标权重:", weights)
```
在上述代码中,我们首先定义了一个3x4的矩阵,表示3个指标对应的4个候选方案的评价值。然后通过除以每列的和来标准化矩阵。接下来,计算每列的熵值,并根据熵值计算权重。最后打印出指标的权重。
注意,这只是一个简单的示例,实际应用中可能需要根据具体情况进行调整和扩展。
相关问题
熵值法Python代码
以下是一个使用熵值法进行多指标决策的示例Python代码:
```python
import numpy as np
def entropy_weight(matrix):
# 数据归一化
m, n = matrix.shape
matrix = matrix / matrix.sum(axis=0)
# 计算熵值
entropy = -np.nansum(matrix * np.log(matrix), axis=0)
# 计算权重
weight = (1 - entropy) / np.sum(1 - entropy)
return weight
# 示例数据
matrix = np.array([[1, 2, 3],
[4, 5, 6],
[7, 8, 9]])
# 调用熵值法函数
weights = entropy_weight(matrix)
print("各指标权重:", weights)
```
在这个示例中,我们首先将数据进行归一化处理,然后计算每个指标的熵值,最后根据熵值计算每个指标的权重。输出结果为各个指标的权重值。
注意:这只是一个简单的示例代码,实际应用中可能需要根据具体情况进行适当的修改和调整。
熵权法python
熵权法是一种多指标决策方法,可以用于解决多个指标的权重确定问题。在熵权法中,每个指标的权重是根据其熵值和信息熵的比值来计算的。熵值越小,表示该指标的信息量越大,对最终决策结果的影响也越大。
在Python中,可以使用scikit-learn库中的entropy模块来计算熵值。以熵权法确定指标权重为例,可以按照以下步骤进行:
1. 导入需要的库:
```
from sklearn.preprocessing import MinMaxScaler
from sklearn.metrics import entropy
import numpy as np
```
2. 准备数据:
```
data = np.array([[1, 2, 3], [4, 5, 6], [7, 8, 9]])
```
3. 对数据进行归一化处理:
```
scaler = MinMaxScaler()
data_norm = scaler.fit_transform(data)
```
4. 计算每个指标的熵值:
```
entropies = []
for i in range(data.shape[1]):
entropies.append(entropy(data_norm[:, i]))
```
5. 计算每个指标的权重:
```
entropies = np.array(entropies)
weights = (1 - entropies / np.sum(entropies))
```
6. 输出指标权重:
```
print(weights)
```
以上就是使用熵权法确定指标权重的Python实现方法。值得注意的是,该方法仅适用于所有指标都是正向指标的情况,如果存在负向指标,则需要进行相应的转换。
阅读全文
相关推荐
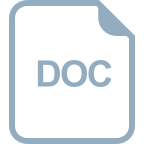
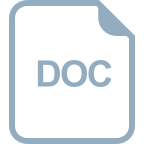
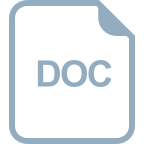
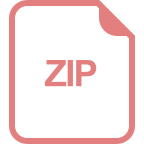
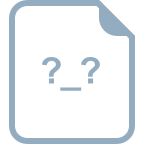











