std::ofstream 用法
时间: 2024-10-17 15:00:57 浏览: 18
`std::ofstream` 是 C++ 标准库中的一个文件流对象,用于将数据写入到文件中。它是 `fstream` 类的一个模板特殊化版本,其中 f 表示“file”,s 表示“stream”。`ofstream` 主要用于向磁盘上创建或追加文本文件写入数据。
以下是使用 `std::ofstream` 的基本步骤:
1. 包含头文件:```#include <fstream>```
2. 创建对象并打开文件:
```cpp
std::ofstream outFile("example.txt"); // 如果文件不存在,会尝试创建
```
3. 检查文件是否成功打开:
```cpp
if (!outFile) {
// 文件打开失败,处理错误
cerr << "Failed to open file." << endl;
}
```
4. 写入数据:
```cpp
outFile << "Hello, World!" << std::endl; // 使用 << 操作符添加内容
```
5. 关闭文件:
```cpp
outFile.close(); // 当不再需要写入时关闭文件
```
注意,`std::ofstream` 提供了异常安全操作,如果你想要在发生错误时立即返回,可以使用 `std::ofstream::exceptions` 构造函数来指定哪种类型的错误会被抛出。
相关问题
[Error] no matching function for call to 'std:basic_ofstream< char>:basic_ofstream(const string&)'
这个错误是由于在创建 `std::ofstream` 对象时传递的参数类型不正确导致的。`std::ofstream` 构造函数需要一个 `const char*` 类型的参数,而你传递的参数是一个 `std::string` 类型。
你可以将 `std::string` 类型的参数转换成 `const char*` 类型,有两种方法可以实现:
1. 使用 `std::string` 对象的 `c_str()` 函数,它返回一个指向字符串的 `const char*` 指针。例如:
```
std::string filename = "file.txt";
std::ofstream outfile(filename.c_str());
```
2. 使用 `std::string` 类型的构造函数,它接受一个 `const char*` 类型的参数。例如:
```
std::string filename = "file.txt";
std::ofstream outfile(filename.data());
```
这两种方法都可以解决这个错误。希望能帮到你!
test.cpp:6:27: error: ‘std::basic_ofstream<char>::__filebuf_type {aka class std::basic_filebuf<char>}’ has no member named ‘fd’ int fd = ofs.rdbuf()->fd();
非常抱歉,我的回答是错误的。在C++标准库中,ofstream类的rdbuf函数确实没有fd方法。如果您需要获取文件描述符,可以使用文件流的native_handle函数来获取文件句柄,然后使用操作系统相关的函数将文件句柄转换为文件描述符,例如在Linux中可以使用fileno函数。
以下是一个示例代码,使用native_handle函数获取文件句柄,并将其转换为文件描述符:
```c++
#include <iostream>
#include <fstream>
#ifdef _WIN32
#include <io.h>
#else
#include <unistd.h>
#endif
int main() {
std::ofstream ofs("test.txt");
int handle = ofs.rdbuf()->native_handle();
#ifdef _WIN32
int fd = _open_osfhandle(handle, 0);
#else
int fd = handle;
#endif
std::cout << "File descriptor: " << fd << std::endl;
ofs << "Hello, world!" << std::endl;
return 0;
}
```
在上面的示例中,通过rdbuf函数获取ofs对象关联的基础流对象的指针,并调用该指针的native_handle函数获取文件句柄。然后,通过操作系统相关的函数将文件句柄转换为文件描述符。最后,在屏幕上输出文件描述符的值。需要注意的是,该代码使用了操作系统相关的函数,因此在不同的平台上可能会有所不同。
阅读全文
相关推荐
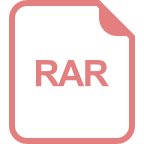
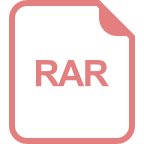
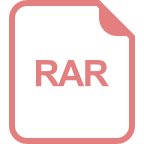









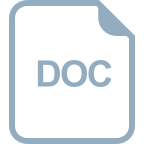
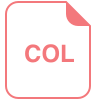
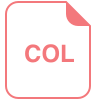
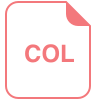
