1,一直监控键盘,键盘里面输入的输入写入到文件a.txt当中 2,如果键盘输入exit,则退出程序,最后打印整个文件的大小 在Linux系统中使用c语言编译
时间: 2024-11-24 20:42:29 浏览: 6
在Linux系统中,使用C语言编写的代码可以按照这样的思路实现:
1. 监控键盘输入并写入文件:
```c
#include <stdio.h>
#include <unistd.h> // 包含unistd.h库用于获取当前进程ID
#include <fcntl.h> // 包含文件描述符操作
#include <sys/ioctl.h> // 包含键盘事件控制
#define FILE_NAME "a.txt"
#define MAX_LINE_LENGTH 1024
void read_keyboard_and_write_to_file(int fd) {
char input[MAX_LINE_LENGTH];
while (true) {
ssize_t bytes_read = read(fd, input, MAX_LINE_LENGTH);
if (bytes_read <= 0) break; // 输入结束或者错误
if (strcmp(input, "exit") == 0) {
break; // 如果输入是"exit",则退出循环
}
write(1, input, bytes_read); // 写入标准输出,即终端
write(fileno(stdout), input, bytes_read); // 同样写入文件
}
}
int main() {
int keyboard_fd = open("/dev/input/event0", O_RDONLY | O_NONBLOCK); // 打开键盘设备
if (keyboard_fd < 0) {
perror("Failed to open keyboard device");
return 1;
}
int file_fd = open(FILE_NAME, O_APPEND | O_CREAT, S_IRUSR | S_IWUSR); // 创建或打开文件
if (file_fd < 0) {
perror("Failed to open file");
close(keyboard_fd);
return 1;
}
read_keyboard_and_write_to_file(keyboard_fd);
close(keyboard_fd);
printf("Closing %s... \n", FILE_NAME);
close(file_fd);
return 0;
}
```
此段代码首先通过`open()`函数打开键盘设备,然后创建一个文件,并在主循环中持续读取键盘输入。当检测到输入为"exit"时,跳出循环并关闭文件。
2. 结束程序并打印文件大小:
```c
#include <fstat.h>
// 在上述main函数结束前添加这行
struct stat file_stat;
fstat(file_fd, &file_stat);
printf("File size: %lld bytes\n", file_stat.st_size);
```
这里使用了`fstat()`函数获取文件的状态信息,包括大小,并将其打印出来。
注意:这个例子假设/dev/input/event0是键盘设备的名称,实际应用中需要根据实际情况调整。此外,对于长时间运行的应用,可能需要处理可能发生的阻塞、异常等问题。
阅读全文
相关推荐
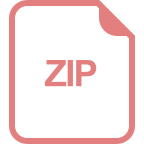
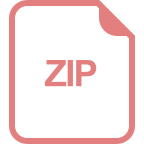
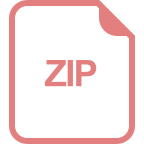

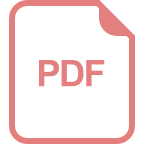
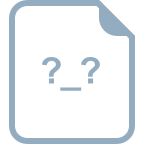
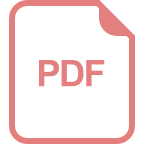
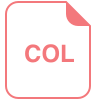
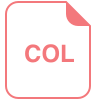
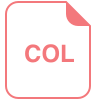
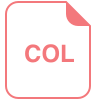
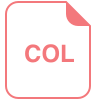
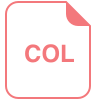
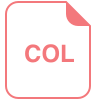
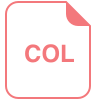
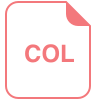
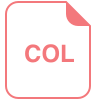
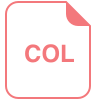