C#Winform文本框的输入值,确保它的输入是数字如何检查
时间: 2024-11-06 22:17:29 浏览: 29
在C# WinForms中,要确保文本框的输入值是数字,可以通过多种方式进行检查。以下是几种常见的方法:
### 1. 使用 `KeyPress` 事件
通过处理 `KeyPress` 事件,可以限制用户只能输入数字和小数点。
```csharp
private void textBox1_KeyPress(object sender, KeyPressEventArgs e)
{
// 允许控制字符(如退格键)
if (char.IsControl(e.KeyChar))
{
return;
}
// 检查是否为数字
if (!char.IsDigit(e.KeyChar) && e.KeyChar != '.')
{
e.Handled = true; // 阻止非数字字符的输入
}
// 防止多个小数点
if (e.KeyChar == '.' && (sender as TextBox).Text.IndexOf('.') > -1)
{
e.Handled = true;
}
}
```
### 2. 使用 `Validating` 事件
在 `Validating` 事件中,可以对整个文本框的内容进行验证。
```csharp
private void textBox1_Validating(object sender, System.ComponentModel.CancelEventArgs e)
{
double result;
if (!double.TryParse((sender as TextBox).Text, out result))
{
MessageBox.Show("请输入有效的数字");
e.Cancel = true; // 取消事件,使焦点保持在文本框中
}
}
```
### 3. 使用正则表达式
可以使用正则表达式来验证输入内容。
```csharp
private void textBox1_TextChanged(object sender, EventArgs e)
{
string pattern = @"^\d+(\.\d+)?$"; // 匹配整数或小数
if (!System.Text.RegularExpressions.Regex.IsMatch((sender as TextBox).Text, pattern))
{
MessageBox.Show("请输入有效的数字");
(sender as TextBox).Clear(); // 清空文本框内容
}
}
```
### 4. 使用 `NumericUpDown` 控件
如果只需要用户输入数字,可以考虑使用 `NumericUpDown` 控件,它内置了数值输入的限制。
```csharp
NumericUpDown numericUpDown1 = new NumericUpDown();
numericUpDown1.Minimum = 0; // 设置最小值
numericUpDown1.Maximum = 100; // 设置最大值
numericUpDown1.Increment = 1; // 设置步长
this.Controls.Add(numericUpDown1); // 添加到窗体中
```
###
阅读全文
相关推荐
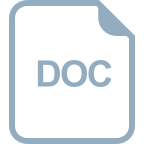
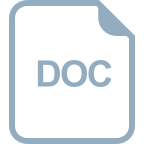
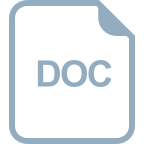

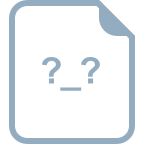
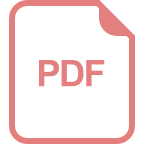
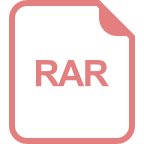
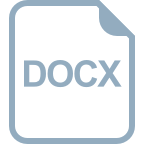



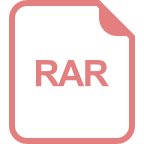
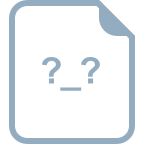
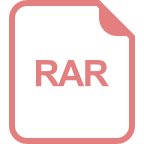
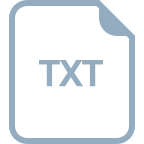
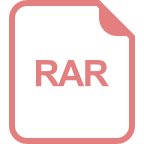


